用c#写一个完整的获取token的代码
时间: 2024-09-07 09:06:24 浏览: 82
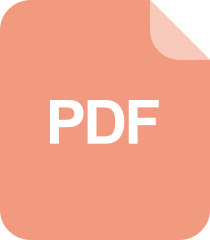
C# wx获取token的基本方法
在C#中获取token通常涉及到身份验证和授权,这可以通过多种方式实现,例如使用OAuth 2.0、JWT(JSON Web Tokens)等协议。以下是一个使用HTTP请求获取token的简单示例代码,假设我们使用的是一个标准的OAuth 2.0认证服务器。
```csharp
using System;
using System.Net.Http;
using System.Text;
using System.Threading.Tasks;
using Newtonsoft.Json.Linq;
public class TokenRetriever
{
private readonly string _clientId;
private readonly string _clientSecret;
private readonly string _tokenEndpoint;
public TokenRetriever(string clientId, string clientSecret, string tokenEndpoint)
{
_clientId = clientId;
_clientSecret = clientSecret;
_tokenEndpoint = tokenEndpoint;
}
public async Task<string> GetTokenAsync()
{
using (var client = new HttpClient())
{
// 构建请求体,根据认证服务器的要求可能有所不同
var values = new FormUrlEncodedContent(new[]
{
new KeyValuePair<string, string>("grant_type", "client_credentials"),
new KeyValuePair<string, string>("client_id", _clientId),
new KeyValuePair<string, string>("client_secret", _clientSecret)
});
// 发送POST请求到认证服务器的token端点
var response = await client.PostAsync(_tokenEndpoint, values);
if (response.IsSuccessStatusCode)
{
// 读取响应内容
var responseContent = await response.Content.ReadAsStringAsync();
// 解析响应内容为JSON
var json = JObject.Parse(responseContent);
// 获取token
var token = json["access_token"]?.ToString();
return token;
}
else
{
// 如果响应失败,抛出异常
throw new Exception("Failed to obtain token");
}
}
}
}
// 使用示例
class Program
{
static async Task Main(string[] args)
{
var retriever = new TokenRetriever("your-client-id", "your-client-secret", "https://your-auth-server.com/token");
try
{
var token = await retriever.GetTokenAsync();
Console.WriteLine($"Access Token: {token}");
}
catch (Exception ex)
{
Console.WriteLine(ex.Message);
}
}
}
```
这个例子中,我们创建了一个`TokenRetriever`类,它有三个属性:`_clientId`(客户端ID)、`_clientSecret`(客户端密钥)和`_tokenEndpoint`(获取token的端点URL)。`GetTokenAsync`方法发送一个POST请求到这个端点,并期望收到一个包含`access_token`的响应。
请注意,上面的代码示例使用了Newtonsoft.Json库来解析JSON响应,你需要通过NuGet安装这个库。另外,错误处理在这个例子中是简化的,实际应用中可能需要更精细的错误处理和重试逻辑。
阅读全文
相关推荐
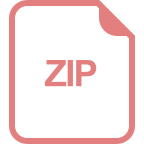
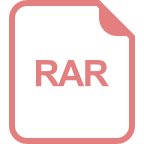















