如何在编程中实现两个集合之间的交集操作并提供一个相关代码示例(用C语言)?
时间: 2024-11-22 07:38:13 浏览: 22
在编程中,实现两个集合之间的交集操作通常涉及到创建一个新的集合,其中包含同时存在于原始两个集合中的元素。这里以C语言为例,由于C语言的标准库并不直接支持集合操作,我们可以借助一些数据结构如数组或链表,并通过循环遍历来实现这个功能。以下是一个简单的示例,使用了数组:
```c
#include <stdio.h>
#include <stdbool.h>
#define MAX_SIZE 100
typedef struct {
int data[MAX_SIZE];
int count;
} Collection;
// 添加元素到集合
void add_element(Collection* collection, int element) {
if (collection->count < MAX_SIZE) {
collection->data[collection->count++] = element;
} else {
printf("Collection is full.\n");
}
}
// 检查元素是否在集合中
bool is_in_collection(const Collection* collection, int element) {
for (int i = 0; i < collection->count; i++) {
if (collection->data[i] == element)
return true;
}
return false;
}
// 交集操作
void intersection(Collection* collection1, Collection* collection2, Collection* result) {
for (int i = 0; i < collection1->count; i++) {
if (is_in_collection(collection2, collection1->data[i])) {
add_element(result, collection1->data[i]);
}
}
}
int main() {
Collection set1, set2, result;
// 初始化集合
set1.count = 5;
set1.data[0] = 1;
set1.data[1] = 2;
set1.data[2] = 3;
set1.data[3] = 4;
set1.data[4] = 5;
set2.count = 4;
set2.data[0] = 2;
set2.data[1] = 3;
set2.data[2] = 5;
set2.data[3] = 6;
// 创建结果集合
result.count = 0;
intersection(&set1, &set2, &result);
printf("Intersection of the two sets:\n");
for (int i = 0; i < result.count; i++) {
printf("%d ", result.data[i]);
}
return 0;
}
```
在这个例子中,`intersection`函数实现了两个集合的交集操作,`main`函数展示了如何使用这个函数。注意这只是一个基础的示例,实际应用中可能会有更复杂的数据结构和算法优化。
阅读全文
相关推荐
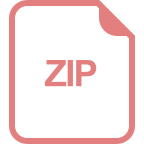
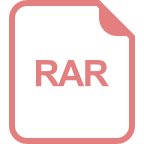
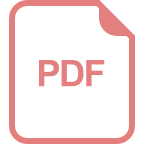
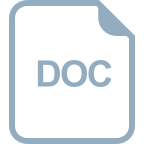




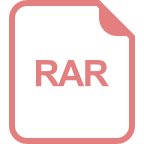
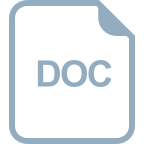
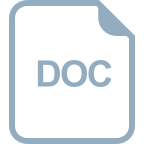
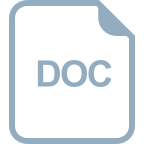
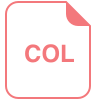
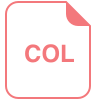
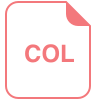
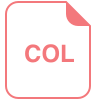
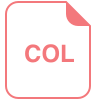
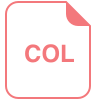
