jps算法c++代码
时间: 2023-10-22 12:29:40 浏览: 137
当然,我可以为您提供JPS算法的C代码示例。下面是一个简单的实现:
```c
#include <stdio.h>
#include <stdlib.h>
#include <stdbool.h>
#define ROWS 10
#define COLS 10
typedef struct {
int x;
int y;
} Point;
typedef struct {
int f;
int g;
int h;
Point parent;
Point current;
} Node;
int heuristic(Point start, Point end) {
return abs(end.x - start.x) + abs(end.y - start.y);
}
bool isValid(int x, int y) {
return (x >= 0 && x < ROWS && y >= 0 && y < COLS);
}
bool isBlocked(int grid[ROWS][COLS], int x, int y) {
return (grid[x][y] == 1);
}
bool isDestination(Point current, Point end) {
return (current.x == end.x && current.y == end.y);
}
void tracePath(Node node, int grid[ROWS][COLS]) {
printf("Path: \n");
while (!(node.parent.x == node.current.x && node.parent.y == node.current.y)) {
printf("(%d, %d)\n", node.current.x, node.current.y);
Point temp = node.parent;
node.parent = node.parent.parent;
node.current = temp;
}
printf("(%d, %d)\n", node.current.x, node.current.y);
}
void jumpPointSearch(int grid[ROWS][COLS], Point start, Point end) {
if (!isValid(start.x, start.y) || !isValid(end.x, end.y)) {
printf("Invalid start or end point.\n");
return;
}
if (isBlocked(grid, start.x, start.y) || isBlocked(grid, end.x, end.y)) {
printf("Start or end point is blocked.\n");
return;
}
bool closedList[ROWS][COLS];
memset(closedList, false, sizeof(closedList));
Node node;
node.f = 0;
node.g = 0;
node.h = 0;
node.parent = start;
node.current = start;
int dx[] = {-1, 1, 0, 0, -1, -1, 1, 1};
int dy[] = {0, 0, -1, 1, -1, 1, -1, 1};
while (!isDestination(node.current, end)) {
int x = node.current.x;
int y = node.current.y;
closedList[x][y] = true;
for (int i = 0; i < 8; i++) {
int newX = x + dx[i];
int newY = y + dy[i];
if (isValid(newX, newY) && !closedList[newX][newY] && !isBlocked(grid, newX, newY)) {
int gNew = node.g + abs(dx[i]) + abs(dy[i]);
int hNew = heuristic({newX, newY}, end);
int fNew = gNew + hNew;
if (grid[newX][newY] == 0 || fNew < grid[newX][newY]) {
grid[newX][newY] = fNew;
Node newNode;
newNode.f = fNew;
newNode.g = gNew;
newNode.h = hNew;
newNode.parent = node.current;
newNode.current = {newX, newY};
node = newNode;
}
}
}
}
tracePath(node, grid);
}
int main() {
int grid[ROWS][COLS] = {
{0, 0, 1, 0, 0, 0, 0, 0, 0, 0},
{0, 0, 1, 0, 0, 0, 0, 0, 0, 0},
{0, 0, 1, 0, 0, 0, 0, 0, 0, 0},
{0, 0, 1, 0, 0, 0, 0, 0, 0, 0},
{0, 0, 1, 0, 1, 1, 1, 1, 1, 1},
{0, 0, 1, 0, 1, 0, 0, 0, 0, 1},
{0, 0, 1, 0, 1, 1, 1, 1, 0, 1},
{0, 0, 1, 1, 1, 1, 1, 1, 0, 1},
{0, 0, 0, 0, 1, 0, 1, 1, 1, 1},
{0, 0, 1 ,1 ,1 ,1 ,1 ,1 ,1 ,1}
};
Point start = {0 ,2};
Point end = {9 ,9};
jumpPointSearch(grid, start ,end);
return 0;
}
```
这是一个简单的网格地图上使用JPS算法寻找路径的示例。请注意,这个示例是针对一个10x10的网格地图,您可以根据需要进行适当的调整。在代码中,0表示可通过的区域,1表示阻塞的区域。算法将打印出从起点到终点的路径坐标。
阅读全文
相关推荐
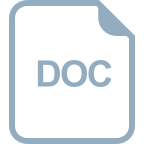
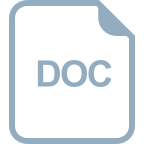
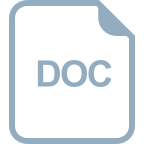



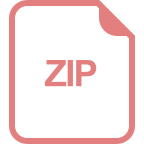
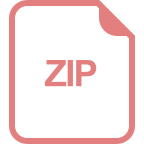
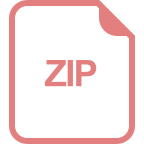
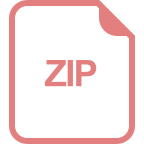
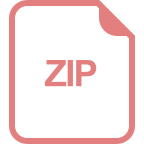
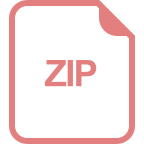
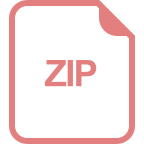
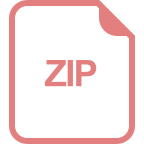
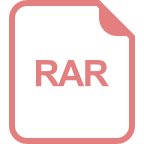
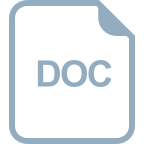
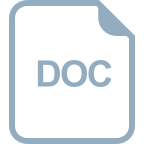
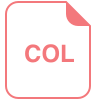
