if __name__ == "__main__": # 测试函数 inp = torch.randn((584, 1, 9, 9)) model = CNN() out = model(inp) print(out.shape)
时间: 2024-05-18 22:12:27 浏览: 136
这段代码是一个测试函数,用于测试一个名为CNN的模型在输入数据inp上的输出结果out的形状shape。其中,torch.randn()是用于生成随机数的函数,inp的形状为(584, 1, 9, 9),表示有584个数据,每个数据有1个通道,每个通道大小为9x9。model(inp)是将inp数据输入到CNN模型中进行计算,得到输出结果out。最后,print(out.shape)是将out的形状输出到控制台上,以便我们查看。
相关问题
def build_transpose(self, layer): in_layout = layer.in_layout out_layout = layer.out_layout align_c = 16 if in_layout == 'NC1HWC0' and out_layout == 'NCHW': in_n = layer.in_shape[0] in_c = layer.in_shape[1] in_h = layer.in_shape[2] in_w = layer.in_shape[3] out_c = layer.out_shape[1] out_h = layer.out_shape[2] out_w = layer.out_shape[3] in_shape = (in_c // align_c, in_h, in_w, align_c) org_out_shape = (out_c, out_h, out_w) elif in_layout == 'NCHW' and out_layout == 'NC2HWC1C0': in_n = layer.in_shape[0] in_c = layer.in_shape[1]*layer.in_shape[2] in_h = layer.in_shape[3] in_w = layer.in_shape[4] out_c2 = layer.out_shape[1] out_c1 = layer.in_shape[2] out_h = layer.out_shape[2] out_w = layer.out_shape[3] in_shape = (in_n, in_c, in_h, in_w) org_out_shape = (out_c2, out_h, out_w, out_c1) input = tvm.placeholder(in_shape, name="input", dtype=env.inp_dtype) #topi with self.m_target: res = top.python.nn.conv2d.transpose(input, org_out_shape, in_layout, out_layout, input.dtype) s = top.python.nn.conv2d.schedule_transpose([res]) #build mod = build_module.build(s, [input, res], target=self.m_target, target_host=env.target_host, name="conv2d") return mod这段是什么意思
这段代码是一个函数,它的作用是构建一个转置卷积操作的模块。该函数包含以下步骤:
1. 获取输入和输出的数据布局(in_layout和out_layout)以及对应的形状(in_shape和out_shape)。
2. 根据不同的布局,计算出输入和输出数据在内存中的存储方式,并对输入数据进行格式转换,以便后续的计算。
3. 使用TVM框架中的topi库,调用python.nn.conv2d.transpose()函数,实现转置卷积操作。
4. 使用TVM的调度器(schedule)对转置卷积操作进行优化和调度。
5. 使用TVM的编译器(build_module)将优化后的操作编译成可执行的模块,以便在特定的硬件上运行。
总之,该函数的作用是根据输入和输出的形状和数据布局构建一个转置卷积操作的模块,以便后续使用。
class DyCAConv(nn.Module): def __init__(self, inp, oup, kernel_size, stride, reduction=32): super(DyCAConv, self).__init__() self.pool_h = nn.AdaptiveAvgPool2d((None, 1)) self.pool_w = nn.AdaptiveAvgPool2d((1, None)) self.pool_h1 = nn.MaxPool2d((None, 1)) self.pool_w1 = nn.MaxPool2d((1, None)) mip = max(8, inp // reduction) self.conv1 = nn.Conv2d(inp, mip, kernel_size=1, stride=1, padding=0) self.bn1 = nn.BatchNorm2d(mip) self.act = h_swish() self.conv_h = nn.Conv2d(mip, inp, kernel_size=1, stride=1, padding=0) self.conv_w = nn.Conv2d(mip, inp, kernel_size=1, stride=1, padding=0) self.conv = nn.Sequential(nn.Conv2d(inp, oup, kernel_size, padding=kernel_size // 2, stride=stride), nn.BatchNorm2d(oup), nn.SiLU()) self.dynamic_weight_fc = nn.Sequential( nn.Linear(inp, 2), nn.Softmax(dim=1) ) def forward(self, x): identity = x n, c, h, w = x.size() x_h = self.pool_h(x) x_w = self.pool_w(x).permute(0, 1, 3, 2) x_h1 = self.pool_h1(x) x_w1 = self.pool_w1(x).permute(0, 1, 3, 2) y = torch.cat([x_h, x_w, x_h1, x_w1], dim=2) y = self.conv1(y) y = self.bn1(y) y = self.act(y) x_h, x_w, _, _ = torch.split(y, [h, w, h, w], dim=2) x_w = x_w.permute(0, 1, 3, 2) x_w1 = x_w1.permute(0, 1, 3, 2) a_h = self.conv_h(x_h).sigmoid() a_w = self.conv_w(x_w).sigmoid() a_w1 = self.conv_w(x_w1).sigmoid() # Compute dynamic weights x_avg_pool = nn.AdaptiveAvgPool2d(1)(x) x_avg_pool = x_avg_pool.view(x.size(0), -1) dynamic_weights = self.dynamic_weight_fc(x_avg_pool) out = identity * (dynamic_weights[:, 0].view(-1, 1, 1, 1) * a_w + dynamic_weights[:, 1].view(-1, 1, 1, 1) * a_h + dynamic_weights[:, 1].view(-1, 1, 1, 1) * a_w1) return self.conv(out)在里面修改一下,换成这个y = torch.cat([x_h+x_h1, x_w+x_w1], dim=2)
如果您想将原来代码中的y拼接成x_h+x_h1和x_w+x_w1,可以按照以下方式进行修改:
```
# 将原来的y拆分成x_h, x_w, x_h1, x_w1
x_h, x_w, x_h1, x_w1 = torch.split(y, [h, w, h, w], dim=2)
# 将x_h和x_h1相加,x_w和x_w1相加
x_h = x_h + x_h1
x_w = x_w + x_w1
# 将x_h和x_w拼接起来
y = torch.cat([x_h, x_w], dim=2)
```
这样就可以将x_h和x_h1相加,x_w和x_w1相加,并将它们拼接起来,作为新的y输出了。
阅读全文
相关推荐
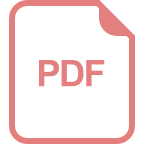
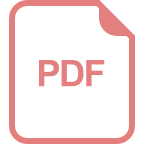













