java打地鼠游戏_用Java实现打地鼠游戏(简版)代码
时间: 2023-12-09 15:04:02 浏览: 39
好的,以下是用Java实现打地鼠游戏(简版)的代码示例:
```java
import javax.swing.*;
import java.awt.*;
import java.awt.event.ActionEvent;
import java.awt.event.ActionListener;
import java.util.Random;
public class WhackAMoleGame extends JFrame implements ActionListener {
private JButton[][] buttons;
private int score;
private JLabel scoreLabel;
private Timer timer;
private Random random;
public WhackAMoleGame() {
super("打地鼠游戏");
// 创建按钮网格布局
JPanel panel = new JPanel(new GridLayout(3, 3));
buttons = new JButton[3][3];
for (int i = 0; i < 3; i++) {
for (int j = 0; j < 3; j++) {
buttons[i][j] = new JButton("地鼠");
buttons[i][j].addActionListener(this);
panel.add(buttons[i][j]);
}
}
add(panel, BorderLayout.CENTER);
// 创建得分标签
score = 0;
scoreLabel = new JLabel("得分:" + score);
add(scoreLabel, BorderLayout.NORTH);
// 创建计时器和随机数生成器
timer = new Timer(1000, this);
random = new Random();
}
@Override
public void actionPerformed(ActionEvent e) {
if (e.getSource() instanceof JButton) {
JButton button = (JButton) e.getSource();
if (button.getText().equals("地鼠")) {
score++;
scoreLabel.setText("得分:" + score);
JOptionPane.showMessageDialog(this, "恭喜你,打到了地鼠!");
} else {
JOptionPane.showMessageDialog(this, "很遗憾,你没有打到地鼠");
}
} else if (e.getSource() == timer) {
// 随机显示地鼠
int row = random.nextInt(3);
int col = random.nextInt(3);
buttons[row][col].setText("地鼠");
// 2秒后隐藏地鼠
timer.setInitialDelay(2000);
buttons[row][col].setText("");
}
}
public static void main(String[] args) {
WhackAMoleGame game = new WhackAMoleGame();
game.setSize(300, 300);
game.setDefaultCloseOperation(JFrame.EXIT_ON_CLOSE);
game.setVisible(true);
game.timer.start();
}
}
```
希望这个代码示例可以帮助您更好地理解如何用Java实现打地鼠游戏。
相关推荐
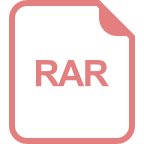














