cv::imshow("video", *frame);
时间: 2024-06-07 08:10:01 浏览: 76
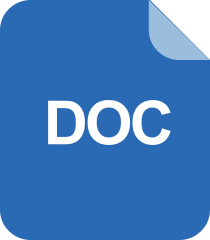
使用Opencv打开处理视频文件
这段代码使用OpenCV库中的imshow函数来展示图像或视频帧。其中,第一个参数是窗口名称,第二个参数是指向图像或视频帧的指针。如果你在程序中读取视频文件,那么这个指针就是从视频中读取到的帧。如果你在程序中捕捉摄像头,则需要使用cv::VideoCapture类来捕捉摄像头帧,然后将其传递给imshow函数。请注意,imshow函数只能在图形界面中运行的情况下使用,如果你在命令行中运行程序,则无法显示图像。
阅读全文
相关推荐
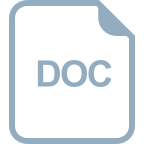
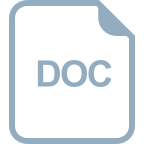





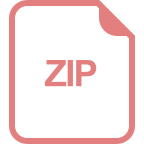
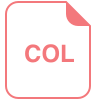









