python使用opencv车牌识别
时间: 2023-10-11 07:12:44 浏览: 51
要使用Python和OpenCV进行车牌识别,需要完成以下步骤:
1. 安装OpenCV库。可以使用pip命令在终端或命令提示符中安装,例如:
```
pip install opencv-python
```
2. 加载图像。可以使用OpenCV的`imread`函数加载图像,例如:
```python
import cv2
image = cv2.imread('car.jpg')
```
3. 对图像进行预处理。可以使用一些技术来增强图像,例如灰度化、二值化、滤波等。以下是一个例子:
```python
gray = cv2.cvtColor(image, cv2.COLOR_BGR2GRAY)
blur = cv2.GaussianBlur(gray, (5, 5), 0)
thresh = cv2.threshold(blur, 0, 255, cv2.THRESH_BINARY_INV + cv2.THRESH_OTSU)[1]
```
4. 找到车牌区域。可以使用形态学操作和边缘检测技术来找到车牌的轮廓,例如:
```python
kernel = cv2.getStructuringElement(cv2.MORPH_RECT, (3,3))
dilate = cv2.dilate(thresh, kernel, iterations=4)
contours, hierarchy = cv2.findContours(dilate, cv2.RETR_EXTERNAL, cv2.CHAIN_APPROX_SIMPLE)
contours = sorted(contours, key=cv2.contourArea, reverse=True)[:10]
```
5. 对车牌区域进行OCR识别。可以使用第三方OCR库,例如Tesseract,对车牌区域进行识别,例如:
```python
import pytesseract
for contour in contours:
x, y, w, h = cv2.boundingRect(contour)
roi = image[y:y+h, x:x+w]
text = pytesseract.image_to_string(roi, lang='eng', config='--psm 7')
print(text)
```
这就是使用Python和OpenCV进行车牌识别的基本步骤。当然,具体实现还要根据不同情况进行调整和优化。
相关推荐
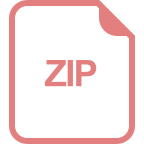
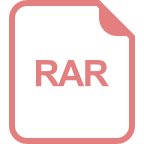
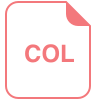
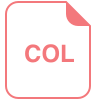
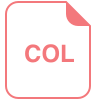
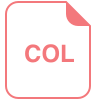
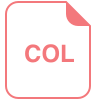








