mongoTemplate 列表查询 指定字段
时间: 2023-10-06 11:09:12 浏览: 90
如果你使用Spring中的MongoTemplate进行查询,可以使用以下方式指定查询字段:
```java
Query query = new Query();
query.fields().include("field1").include("field2");
List<Document> results = mongoTemplate.find(query, Document.class, "collectionName");
```
在这个例子中,我们创建了一个查询对象`query`,并通过`query.fields()`方法指定要包含的字段。在这个例子中,我们指定了`field1`和`field2`两个字段。最后,我们使用`mongoTemplate.find()`方法执行查询,指定要查询的集合名称和返回的文档类型。
如果你想查询所有字段除了指定的字段,可以使用`exclude()`方法。例如:
```java
query.fields().exclude("field3");
```
这将查询所有字段除了`field3`字段。
相关问题
mongotemplate返回指定字段
### 回答1:
使用Mongotemplate返回指定字段,可以使用Mongotemplate中的project方法。在项目方法中,可以使用ProjectionOperation来指定要返回的字段。例如:
Query query = new Query();
query.addCriteria(Criteria.where("name").is("张三"));
ProjectionOperation project = Aggregation.project("name", "age");
Aggregation aggregation = Aggregation.newAggregation(project);
List<Map<String,Object>> result = mongoTemplate.aggregate(aggregation, collectionName, Map.class).getMappedResults();
这个查询将返回名字和年龄在数据库中为“张三”的人的信息。
### 回答2:
Mongotemplate是Spring Data MongoDB提供的一个核心类,它为我们封装了MongoDB的操作方法,让我们可以方便地进行CRUD操作。Mongotemplate返回指定字段的方法是通过使用Projection来实现的。
Projection是MongoDB的一个特性,它可以指定查询结果文档中需要返回的字段。在Mongotemplate中,我们可以使用Query对象的fields方法指定Projection,将需要返回的字段加入到Projection中。
例如,我们有一个Person实体类,它有id、name、age、gender、address等属性。我们可以使用如下代码查询并返回只包含id和name的Person数据:
```
Query query = new Query();
query.fields().include("id", "name");
List<Person> persons = mongoTemplate.find(query, Person.class);
```
在这个例子中,我们通过Query对象的fields方法指定Projection,使用include方法将需要返回的字段id和name加入到Projection中。然后,使用mongoTemplate的find方法查询数据,并将返回结果转换为Person类的List对象。
除了使用include方法指定需要返回的字段之外,我们还可以使用exclude方法指定不需要返回的字段,也可以使用elemMatch方法指定查询嵌套文档字段的Projection。
总的来说,Mongotemplate返回指定字段的方法就是通过Projection来实现的,即使用Query对象的fields方法指定Projection,将需要返回的字段加入到Projection中。在实际开发中,我们可以根据具体的业务需求来选择合适的Projection方法,以达到优化查询性能、提高查询效率的目的。
### 回答3:
MongoTemplate是Spring Data MongoDB提供的一种操作MongoDB的工具,它提供了一系列方法可以方便地对MongoDB进行操作。通过MongoTemplate返回指定字段需要通过指定查询条件和投影条件来实现。
查询条件是指MongoDB中的查询条件,它可以是一个满足MongoDB语法的查询条件对象或文档。投影条件是指查询结果中应该包含的字段。MongoDB的投影操作可以利用字典文档的形式来指定需要返回的字段。MongoTemplate中提供了projection方法来实现这一功能,它需要传入一个ProjectionOperation对象用来指定需要返回的字段。
ProjectionOperation是一个MongoDB Projection操作的工具类,它提供了一些方法用来构造ProjectionOperation对象。其中,include和exclude方法可以指定需要返回或不返回的字段。当指定需要返回的字段时,可以使用不同的方式来指定:include(“fieldName”)用来指定单个字段,include(fields…)用来指定多个字段。当指定不需要返回的字段时,exclude(“fieldName”)用来指定单个字段,exclude(“fieldName1”, “fieldName2”)用来指定多个字段。
最后,可以通过MongoTemplate的find方法来执行查询,该方法需要传入一个Query对象和一个Class<T>类型的参数。Query对象用来指定查询条件,Class<T>类型的参数用来指定查询结果的类型。当指定了ProjectionOperation对象后,查询结果将会按照投影条件中的字段返回,而不是返回所有字段。
综上所述,通过MongoTemplate返回指定字段需要完成以下步骤:
1. 构造查询条件和投影条件;
2. 使用ProjectionOperation对象指定需要返回的字段;
3. 使用find方法执行查询,结果将按照投影条件中的字段返回。
springBoot mongoTemplate查询mongo数组字段中匹配的数据
### 回答1:
在 Spring Boot 中使用 MongoTemplate 查询 MongoDB 数组字段中匹配特定值的数据,可以使用 `Query` 类来构建查询。
具体实现方法如下:
```java
Query query = new Query(Criteria.where("arrayFieldName").is("valueToMatch"));
List<YourEntity> results = mongoTemplate.find(query, YourEntity.class);
```
其中 `arrayFieldName` 是要查询的数组字段名称,`valueToMatch` 是要匹配的值,`YourEntity` 是数据实体类。
如果你要查询数组字段包含特定值的数据,你可以使用 `Criteria.where("arrayFieldName").in("valueToMatch")`
```java
Query query = new Query(Criteria.where("arrayFieldName").in("valueToMatch"));
List<YourEntity> results = mongoTemplate.find(query, YourEntity.class);
```
如果你要在数组字段中查询一些特定值,可以使用 $elemMatch
```java
Query query = new Query(Criteria.where("arrayFieldName").elemMatch(Criteria.where("fieldName").is("valueToMatch")));
List<YourEntity> results = mongoTemplate.find(query, YourEntity.class);
```
以上是最简单的查询方法,MongoTemplate支持多种条件查询,你可以根据需要调整查询语句,以获取更精确的结果.
### 回答2:
在Spring Boot中,我们可以使用MongoTemplate来查询MongoDB中数组字段中匹配的数据。
首先,在我们的实体类中定义一个数组字段,例如:
```java
@Document(collection = "example")
public class ExampleEntity {
// 其他字段...
private List<String> fruits;
// 构造函数、getter和setter...
}
```
然后,在我们的数据访问层或服务层中,使用MongoTemplate来查询包含特定值的数组字段。
```java
@Autowired
private MongoTemplate mongoTemplate;
public List<ExampleEntity> findMatchingFruits(String fruit) {
Query query = new Query(Criteria.where("fruits").in(fruit));
return mongoTemplate.find(query, ExampleEntity.class);
}
```
在上面的例子中,我们通过传入特定的水果名称来查询包含该水果名称的数组字段数据。使用Criteria的in方法可以匹配数组字段中的一个或多个值。
最后,我们可以在需要的地方调用该方法来查询匹配的数据。
```java
List<ExampleEntity> matchingFruits = exampleService.findMatchingFruits("苹果");
```
这样,我们就可以使用Spring Boot和MongoTemplate来查询MongoDB中数组字段中匹配的数据。
### 回答3:
若要使用Spring Boot中的`MongoTemplate`查询MongoDB数组字段中匹配的数据,可以采用以下步骤:
1. 确保已经正确配置了MongoDB的连接信息,并且已经注入了`MongoTemplate`实例。
2. 使用`Criteria`对象来构建查询条件,`Criteria`是MongoDB中用于查询的对象。
3. 使用`Criteria.where`方法来指定要查询的字段,以及字段中的条件。例如,假设我们要查询名为`students`的集合中,`scores`数组字段中分数大于80的文档,我们可以使用以下代码:
```java
Criteria criteria = Criteria.where("scores").elemMatch(Criteria.where("score").gt(80));
Query query = Query.query(criteria);
```
4. 使用`mongoTemplate.find`方法来执行查询,并将结果保存在一个`List`对象中。例如:
```java
List<Document> results = mongoTemplate.find(query, Document.class, "students");
```
其中,`students`为集合名,`Document.class`为返回结果的类型。
5. 可以使用`results`对象进行进一步的处理,例如遍历结果集并输出。例如:
```java
for (Document result : results) {
System.out.println(result.toJson());
}
```
以上就是使用Spring Boot中的`MongoTemplate`查询MongoDB数组字段中匹配数据的步骤。具体查询条件和返回结果类型可以根据实际需求进行修改和调整。
相关推荐
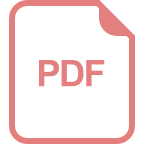












