mongoTemplate联表查询排序
时间: 2023-05-27 18:01:26 浏览: 113
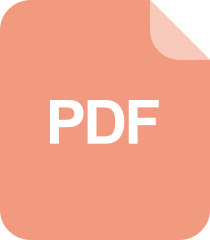
排序查询
1. 使用mongoTemplate进行联表查询
MongoDB中使用$lookup运算符实现联表查询。在Spring Data MongoDB中,可以通过Aggregation类来构建复杂的聚合查询。
例如,假设有两个集合:orders和products,它们的文档结构分别如下:
orders
{
"orderId": 1,
"productId": 1,
"quantity": 5
},
{
"orderId": 2,
"productId": 2,
"quantity": 3
}
products
{
"productId": 1,
"name": "iPhone",
"price": 999
},
{
"productId": 2,
"name": "iPad",
"price": 799
}
现在需要通过orderId查询订单详情,并将订单详情和商品信息联合在一起。可以使用以下代码实现:
// 构建Aggregation查询
Aggregation agg = newAggregation(
match(Criteria.where("orderId").is(orderId)), // 匹配订单ID
lookup("products", "productId", "productId", "product"), // 联表查询
unwind("product"), // 展开查询结果
project("orderId", "quantity", "product.name", "product.price") // 投影查询结果
);
// 执行查询
AggregationResults<OrderDetail> results = mongoTemplate.aggregate(agg, "orders", OrderDetail.class);
其中OrderDetail是一个包含订单详情和商品信息的DTO类:
public class OrderDetail {
private Integer orderId;
private Integer quantity;
private String productName;
private Double productPrice;
// getter和setter方法
}
2. 使用mongoTemplate对查询结果排序
在上述联表查询的基础上,可以通过以下代码对查询结果按照商品价格进行升序排序:
Aggregation agg = newAggregation(
match(Criteria.where("orderId").is(orderId)),
lookup("products", "productId", "productId", "product"),
unwind("product"),
project("orderId", "quantity", "product.name", "product.price"),
sort(Sort.Direction.ASC, "product.price") // 排序
);
AggregationResults<OrderDetail> results = mongoTemplate.aggregate(agg, "orders", OrderDetail.class);
其中sort方法用来指定排序方式,第一个参数为排序方向,第二个参数为排序字段。在这里,我们按照product.price字段进行升序排序。
阅读全文
相关推荐
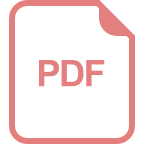
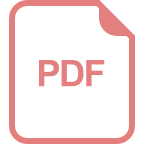













