利用opencv打开摄像头实时测量物体的尺寸
时间: 2023-08-08 12:12:52 浏览: 100
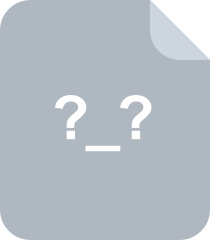
使用opencv打开摄像头
利用OpenCV打开摄像头实时测量物体的尺寸可以通过以下步骤实现:
1. 打开摄像头并获取视频流。
```python
import cv2
cap = cv2.VideoCapture(0) # 0代表第一个摄像头
```
2. 从视频流中读取一帧图像。
```python
ret, frame = cap.read()
```
3. 对图像进行处理,例如转换为灰度图像、平滑处理、边缘检测等。
```python
gray = cv2.cvtColor(frame, cv2.COLOR_BGR2GRAY)
blurred = cv2.GaussianBlur(gray, (5, 5), 0)
edged = cv2.Canny(blurred, 50, 150)
```
4. 对处理后的图像进行轮廓检测。
```python
contours, hierarchy = cv2.findContours(edged, cv2.RETR_LIST, cv2.CHAIN_APPROX_SIMPLE)
```
5. 遍历轮廓,计算物体的尺寸。
```python
for contour in contours:
perimeter = cv2.arcLength(contour, True)
approx = cv2.approxPolyDP(contour, 0.02 * perimeter, True)
if len(approx) == 4: # 假设物体是一个四边形
x, y, w, h = cv2.boundingRect(contour)
cv2.rectangle(frame, (x, y), (x + w, y + h), (0, 255, 0), 2)
cv2.putText(frame, "Object Size: {} x {}".format(w, h), (x, y - 10), cv2.FONT_HERSHEY_SIMPLEX, 0.5, (0, 255, 0), 2)
```
6. 显示处理后的图像。
```python
cv2.imshow("Frame", frame)
```
完整的代码如下:
```python
import cv2
cap = cv2.VideoCapture(0)
while True:
ret, frame = cap.read()
gray = cv2.cvtColor(frame, cv2.COLOR_BGR2GRAY)
blurred = cv2.GaussianBlur(gray, (5, 5), 0)
edged = cv2.Canny(blurred, 50, 150)
contours, hierarchy = cv2.findContours(edged, cv2.RETR_LIST, cv2.CHAIN_APPROX_SIMPLE)
for contour in contours:
perimeter = cv2.arcLength(contour, True)
approx = cv2.approxPolyDP(contour, 0.02 * perimeter, True)
if len(approx) == 4:
x, y, w, h = cv2.boundingRect(contour)
cv2.rectangle(frame, (x, y), (x + w, y + h), (0, 255, 0), 2)
cv2.putText(frame, "Object Size: {} x {}".format(w, h), (x, y - 10), cv2.FONT_HERSHEY_SIMPLEX, 0.5, (0, 255, 0), 2)
cv2.imshow("Frame", frame)
if cv2.waitKey(1) & 0xFF == ord('q'):
break
cap.release()
cv2.destroyAllWindows()
```
在这个例子中,我们假设物体是一个四边形,通过计算其包围矩形的宽度和高度,来估算物体的尺寸。但实际应用中,需要根据具体的场景来选择合适的算法和方法。
阅读全文
相关推荐


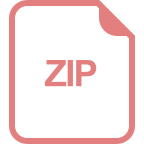
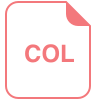







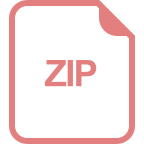
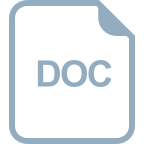
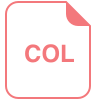
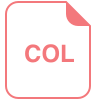
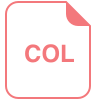