用python写一个自注意力机制的代码
时间: 2023-08-31 08:43:26 浏览: 109
### 回答1:
我们可以使用TensorFlow的Keras API来实现自注意力机制。具体来说,我们可以定义一个Keras模型,其中包含一个自注意力层,并将其与其他层连接起来。下面是一个简单的Python代码示例:import tensorflow as tf
from tensorflow.keras import layers# 定义输入和上下文
inputs = tf.keras.Input(shape=(64,))
context = tf.keras.Input(shape=(64,))# 将输入和上下文连接到自注意力层
attention_layer = layers.Attention()([inputs, context])# 构建模型
model = tf.keras.Model([inputs, context], attention_layer)
model.compile(optimizer=tf.keras.optimizers.Adam(0.01),
loss=tf.keras.losses.CategoricalCrossentropy(from_logits=True),
metrics=['accuracy'])
### 回答2:
自注意力机制是一种被广泛应用于自然语言处理和机器翻译等任务的机制。它可以帮助模型在处理序列数据时更好地捕捉序列之间的关系。下面是使用Python实现自注意力机制的代码示例:
```python
import math
import torch
import torch.nn as nn
class SelfAttention(nn.Module):
def __init__(self, hidden_dim):
super(SelfAttention, self).__init__()
self.hidden_dim = hidden_dim
self.query_fc = nn.Linear(hidden_dim, hidden_dim)
self.key_fc = nn.Linear(hidden_dim, hidden_dim)
self.value_fc = nn.Linear(hidden_dim, hidden_dim)
def forward(self, inputs):
batch_size, seq_len, _ = inputs.size()
queries = self.query_fc(inputs)
keys = self.key_fc(inputs)
values = self.value_fc(inputs)
attn_scores = torch.bmm(queries, keys.transpose(1, 2))
attn_scores = attn_scores / math.sqrt(self.hidden_dim)
attn_probs = torch.softmax(attn_scores, dim=2)
attended_vals = torch.bmm(attn_probs, values)
return attended_vals
# 测试代码
hidden_dim = 128
seq_len = 10
batch_size = 16
inputs = torch.randn(batch_size, seq_len, hidden_dim)
attention = SelfAttention(hidden_dim)
results = attention(inputs)
print(results.size()) # 打印输出结果的形状
```
上述代码实现了一个简单的自注意力机制。在`SelfAttention`类的`forward`函数中,首先通过三个线性层将输入映射到查询(queries)、键(keys)和值(values)的空间。然后通过计算查询和键之间的内积,得到注意力得分矩阵。通过对注意力得分进行缩放(除以根号隐藏维度),并使用softmax函数计算得到注意力权重。最后,利用注意力权重对值进行加权求和,得到最终的自注意力输出。
测试代码中,首先定义了输入的形状,并初始化了一个自注意力模型。然后将输入传入模型,得到输出结果的形状并打印输出。
相关推荐
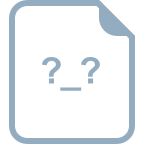
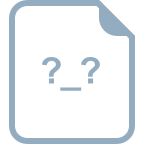














