import copy
时间: 2023-08-26 21:04:55 浏览: 98
The `copy` module in Python provides functions for creating copies of objects. The `copy()` function creates a shallow copy of an object, while the `deepcopy()` function creates a deep copy.
A shallow copy creates a new object with the same values as the original object, but any mutable objects within the original object are still references to the original object. In other words, changes to the mutable objects in the copy will also be reflected in the original object.
A deep copy creates a new object with the same values as the original object, and any mutable objects within the original object are also copied recursively, so changes to the mutable objects in the copy will not be reflected in the original object.
Here is an example to illustrate the difference between shallow copy and deep copy:
```
import copy
# create a list with a nested list
original_list = [1, 2, [3, 4]]
shallow_copy = copy.copy(original_list)
deep_copy = copy.deepcopy(original_list)
# modify the nested list in the shallow copy
shallow_copy[2][0] = 5
# print the original list and the shallow copy
print(original_list) # [1, 2, [5, 4]]
print(shallow_copy) # [1, 2, [5, 4]]
# modify the nested list in the deep copy
deep_copy[2][0] = 6
# print the original list and the deep copy
print(original_list) # [1, 2, [5, 4]]
print(deep_copy) # [1, 2, [6, 4]]
```
In this example, we create a list with a nested list `[3, 4]`. We then create a shallow copy of the original list using `copy.copy()`, and a deep copy using `copy.deepcopy()`. We modify the nested list in the shallow copy by changing the first element from 3 to 5, and we modify the nested list in the deep copy by changing the first element from 3 to 6.
When we print the original list and the shallow copy, we see that the nested list has been modified in both, because the shallow copy only creates a new reference to the nested list. However, when we print the original list and the deep copy, we see that the nested list has only been modified in the deep copy, because the deep copy creates a new copy of the nested list.
阅读全文
相关推荐
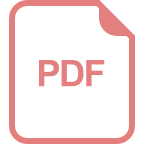
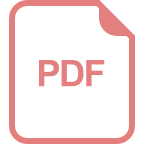















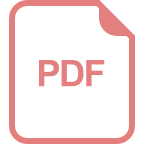