算术表达式求值。演示程序和源程序已给出。用户从键盘输入一个算术表达式,数字都是十进制整型(必须支持负数),运算符支持+-*/()。 编写源程序中的 double deal_exp (char *exp) 函数,对这个算术表达式求值,返回结果。不限制方法,你可以用“原始”的多遍扫描字符串,也可以用先进一点的栈
时间: 2023-12-14 08:38:36 浏览: 112
以下是使用栈实现算术表达式求值的代码示例:
```c++
#include <iostream>
#include <stack>
#include <string>
using namespace std;
// 判断字符是否为数字
bool is_digit(char c) {
return c >= '0' && c <= '9';
}
// 将字符转换成数字
int char_to_digit(char c) {
return c - '0';
}
// 计算两个数字的运算结果
double calculate(double a, double b, char op) {
switch (op) {
case '+':
return a + b;
case '-':
return a - b;
case '*':
return a * b;
case '/':
return a / b;
default:
return 0.0;
}
}
// 处理算术表达式
double deal_exp(char *exp) {
stack<double> nums; // 存放数字的栈
stack<char> ops; // 存放运算符的栈
for (int i = 0; exp[i] != '\0'; i++) {
if (is_digit(exp[i])) { // 如果当前字符是数字,则将其转换成数字并入栈
int num = char_to_digit(exp[i]);
i++;
while (is_digit(exp[i])) {
num = num * 10 + char_to_digit(exp[i]);
i++;
}
i--;
nums.push(num);
} else if (exp[i] == '(') { // 如果当前字符是左括号,则将其入栈
ops.push(exp[i]);
} else if (exp[i] == ')') { // 如果当前字符是右括号,则进行计算直到遇到左括号
while (ops.top() != '(') {
double b = nums.top();
nums.pop();
double a = nums.top();
nums.pop();
char op = ops.top();
ops.pop();
nums.push(calculate(a, b, op));
}
ops.pop();
} else if (exp[i] == '+' || exp[i] == '-') { // 如果当前字符是加减号
while (!ops.empty() && ops.top() != '(') { // 如果栈顶元素是乘除号,则进行计算
double b = nums.top();
nums.pop();
double a = nums.top();
nums.pop();
char op = ops.top();
ops.pop();
nums.push(calculate(a, b, op));
}
ops.push(exp[i]);
} else if (exp[i] == '*' || exp[i] == '/') { // 如果当前字符是乘除号
while (!ops.empty() && (ops.top() == '*' || ops.top() == '/')) { // 如果栈顶元素是乘除号,则进行计算
double b = nums.top();
nums.pop();
double a = nums.top();
nums.pop();
char op = ops.top();
ops.pop();
nums.push(calculate(a, b, op));
}
ops.push(exp[i]);
}
}
while (!ops.empty()) { // 处理剩余的运算符和数字
double b = nums.top();
nums.pop();
double a = nums.top();
nums.pop();
char op = ops.top();
ops.pop();
nums.push(calculate(a, b, op));
}
return nums.top();
}
int main() {
char exp[100];
cout << "请输入算术表达式:";
cin >> exp;
double result = deal_exp(exp);
cout << "计算结果为:" << result << endl;
return 0;
}
```
这个程序使用两个栈分别存放数字和运算符。扫描算术表达式,遇到数字就入数字栈,遇到运算符就和运算符栈顶元素比较优先级,如果当前运算符优先级较高,则入栈;否则,取出数字栈中的两个数字和运算符栈顶元素进行计算,并将结果入数字栈,重复上述操作直到扫描完整个算术表达式。最后,将数字栈中的唯一元素作为计算结果返回。
阅读全文
相关推荐
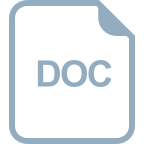




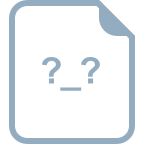
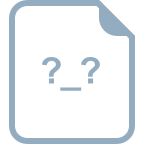
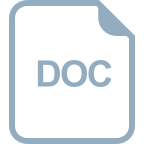
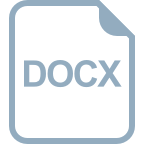
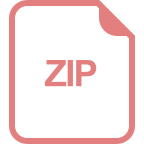
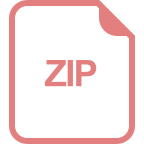
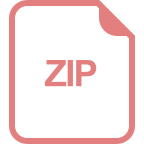
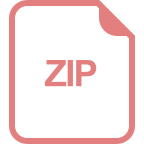