c语言实现at指令驱动模组
时间: 2023-07-26 13:05:35 浏览: 95
AT指令是一种通用的串行接口通信协议,常用于控制模块的操作,例如GSM模块和WiFi模块等。在C语言中,我们可以通过串口通信实现对模块的AT指令控制。
下面是一个简单的C语言代码示例,实现了对GSM模块的AT指令控制:
```c
#include <stdio.h>
#include <string.h>
#include <unistd.h>
#include <fcntl.h>
#include <termios.h>
#define DEVICE "/dev/ttyS0" // 串口设备文件路径
int main()
{
int fd;
struct termios options;
char buf[256];
int len;
fd = open(DEVICE, O_RDWR | O_NOCTTY | O_NDELAY); // 打开串口设备文件
if (fd < 0) {
printf("open %s failed!\n", DEVICE);
return -1;
}
fcntl(fd, F_SETFL, 0); // 阻塞模式
tcgetattr(fd, &options); // 获取串口属性
cfsetispeed(&options, B9600); // 设置波特率为9600
cfsetospeed(&options, B9600);
options.c_cflag |= (CLOCAL | CREAD); // 本地连接和接收使能
options.c_cflag &= ~PARENB; // 无奇偶校验位
options.c_cflag &= ~CSTOPB; // 1位停止位
options.c_cflag &= ~CSIZE; // 数据位长度
options.c_cflag |= CS8;
options.c_lflag &= ~(ICANON | ECHO | ECHOE | ISIG); // 非规范模式
options.c_oflag &= ~OPOST; // 不进行输出处理
tcsetattr(fd, TCSANOW, &options); // 设置串口属性
memset(buf, 0, sizeof(buf));
len = read(fd, buf, sizeof(buf)); // 读取串口数据
if (len > 0) {
printf("%s", buf);
}
sprintf(buf, "AT\r\n");
write(fd, buf, strlen(buf)); // 发送AT指令
sleep(1); // 等待模块响应
memset(buf, 0, sizeof(buf));
len = read(fd, buf, sizeof(buf)); // 读取串口数据
if (len > 0) {
printf("%s", buf);
}
close(fd); // 关闭串口设备文件
return 0;
}
```
以上代码中,我们首先打开了串口设备文件(`/dev/ttyS0`),并通过`fcntl`函数设置阻塞模式。然后,我们通过`tcgetattr`和`cfsetispeed/cfsetospeed`函数获取并设置了串口的属性,包括波特率、数据位长度、停止位、奇偶校验位等。接着,我们发送了一个AT指令,并通过`read`函数读取模块的响应。最后,我们关闭了串口设备文件。
需要注意的是,不同的模块可能支持不同的AT指令集,因此具体的实现方式可能会有所不同。
阅读全文
相关推荐
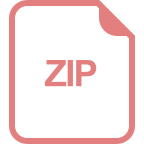
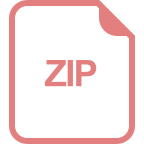
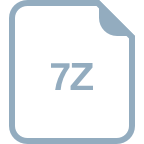















