前端vue3中如何用一个数组接收后端返回的data中的一个数组
时间: 2024-04-30 12:23:22 浏览: 571
可以使用Vue3的响应式数据来接收后端返回的数据。首先,在Vue3中创建一个响应式的数据数组:
```javascript
import { reactive } from 'vue'
const data = reactive({
myArray: []
})
```
然后,在获取后端数据的回调函数中,将后端返回的数组赋值给响应式数据中的数组:
```javascript
axios.get('/api/data').then(response => {
data.myArray = response.data.myArray
})
```
现在,后端返回的数组就可以通过`data.myArray`属性访问并使用了。当数组发生变化时,Vue3会自动更新视图。
相关问题
vue前端传了个String数组 后端如何接收
如果前端使用Vue传递了一个字符串数组,后端可以通过以下方式进行接收:
1. 获取请求体中的参数,并将其转换为数组
```javascript
// Node.js Express 代码示例
const express = require('express');
const bodyParser = require('body-parser');
const app = express();
app.use(bodyParser.json());
app.post('/api/data', (req, res) => {
const data = req.body.data.split(','); // 将逗号分隔的字符串转换为数组
console.log(data);
// do something with data
});
app.listen(3000, () => {
console.log('Server is running at http://localhost:3000');
});
```
在以上代码中,我们首先通过body-parser中间件解析请求体中的JSON数据,并获取到参数`data`的值,该值是一个逗号分隔的字符串。接着,我们使用`split`方法将该字符串转换为数组。
2. 直接将字符串数组作为请求体参数
```javascript
// Node.js Express 代码示例
const express = require('express');
const bodyParser = require('body-parser');
const app = express();
app.use(bodyParser.json());
app.post('/api/data', (req, res) => {
const data = req.body; // 直接获取请求体中的参数
console.log(data);
// do something with data
});
app.listen(3000, () => {
console.log('Server is running at http://localhost:3000');
});
```
在以上代码中,我们直接将请求体中的参数作为一个对象进行获取,该对象包含一个名为`data`的属性,该属性的值是一个字符串数组。后端可以直接使用该数组进行后续操作。
需要注意的是,如果前端传递的是一个JSON字符串,后端需要先将其解析为一个JavaScript对象或数组,才能够进行操作。可以使用`JSON.parse`方法将其转换为对应的JavaScript对象或数组。
vue上传图片和一个数组到python后端,python后端接收图片并下载到本地,python后端使用fastapi,前端使用elementui,前后端的代码怎么写
好的,针对您的问题,我将分别给出前端Vue(使用ElementUI组件库)和后端Python(使用FastAPI框架)的示例代码。
前端Vue代码:
```html
<template>
<div>
<el-upload
ref="upload"
action="/api/upload"
:show-file-list="false"
:on-success="handleSuccess"
:before-upload="beforeUpload"
:data="{ arr: arr }"
>
<el-button>点击上传</el-button>
</el-upload>
</div>
</template>
<script>
import axios from 'axios';
export default {
data() {
return {
arr: [1, 2, 3, 4, 5],
};
},
methods: {
handleSuccess(response) {
console.log(response);
},
beforeUpload(file) {
const formData = new FormData();
formData.append('file', file);
formData.append('arr', JSON.stringify(this.arr));
this.$refs.upload.formData = formData;
},
},
};
</script>
```
上面的代码中,我们使用了`<el-upload>`组件来选择文件并上传,同时将数组作为请求体中的数据进行上传。
后端Python代码:
```python
from fastapi import FastAPI, File, UploadFile, Form
from fastapi.responses import JSONResponse
import os
app = FastAPI()
@app.post("/api/upload")
async def upload(file: UploadFile = File(...), arr: str = Form(...)):
arr = json.loads(arr)
filename = file.filename
with open(os.path.join('/path/to/save/', filename), 'wb') as f:
contents = await file.read()
f.write(contents)
return JSONResponse(content={'msg': 'success'})
if __name__ == '__main__':
import uvicorn
uvicorn.run(app, host="0.0.0.0", port=8000)
```
上面的代码中,我们使用了FastAPI框架来创建 Web 应用,接收前端发送的POST请求,从中获取文件和数组,然后将文件保存到本地指定路径。请注意,`/path/to/save/`需要替换为您本地存储文件的路径。同时,我们使用了`JSONResponse`类来返回JSON格式的响应结果。
需要注意的是,我们在前端使用了ElementUI组件库的`<el-upload>`组件来进行文件上传,同时在`beforeUpload`方法中将数组作为请求体中的数据添加到了`FormData`对象中,并将该对象赋值给了上传组件的`formData`属性。
阅读全文
相关推荐
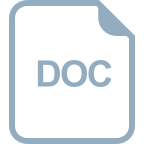
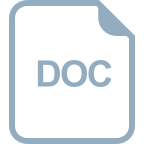
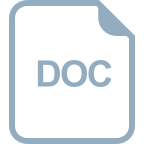



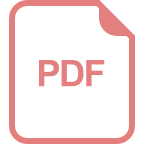










