xdma端口windows例子程序
时间: 2024-02-01 17:14:04 浏览: 21
以下是一个简单的 XDMA 端口 Windows 例子程序,它使用 OpenCL API 进行数据传输:
```c++
#include <CL/cl.h>
#include <stdio.h>
#include <stdlib.h>
#define DATA_SIZE 1024
const char *KernelSource = "\n" \
"__kernel void square( \n" \
" __global float* input, \n" \
" __global float* output, \n" \
" const unsigned int count) \n" \
"{ \n" \
" int i = get_global_id(0); \n" \
" if(i < count) \n" \
" output[i] = input[i] * input[i]; \n" \
"} \n" \
"\n";
int main()
{
cl_context context = NULL;
cl_command_queue commandQueue = NULL;
cl_program program = NULL;
cl_kernel kernel = NULL;
cl_mem input = NULL;
cl_mem output = NULL;
cl_int errNum;
float inputData[DATA_SIZE];
float results[DATA_SIZE];
unsigned int correct;
for(int i = 0; i < DATA_SIZE; i++)
inputData[i] = i;
// 创建 OpenCL 上下文
context = clCreateContextFromType(NULL, CL_DEVICE_TYPE_GPU, NULL, NULL, &errNum);
if(errNum != CL_SUCCESS)
{
printf("Failed to create an OpenCL GPU context.\n");
return 1;
}
// 获取 GPU 设备 ID
size_t deviceBufferSize = -1;
clGetContextInfo(context, CL_CONTEXT_DEVICES, 0, NULL, &deviceBufferSize);
if(deviceBufferSize <= 0)
{
printf("No OpenCL compatible GPU devices found.\n");
return 1;
}
cl_device_id *deviceIDs = (cl_device_id *)malloc(deviceBufferSize);
clGetContextInfo(context, CL_CONTEXT_DEVICES, deviceBufferSize, deviceIDs, NULL);
// 创建命令队列
commandQueue = clCreateCommandQueue(context, deviceIDs[0], 0, NULL);
if(commandQueue == NULL)
{
printf("Failed to create an OpenCL command queue.\n");
return 1;
}
// 创建输入和输出缓冲区
input = clCreateBuffer(context, CL_MEM_READ_ONLY | CL_MEM_COPY_HOST_PTR, sizeof(float) * DATA_SIZE, inputData, NULL);
output = clCreateBuffer(context, CL_MEM_WRITE_ONLY, sizeof(float) * DATA_SIZE, NULL, NULL);
if(input == NULL || output == NULL)
{
printf("Failed to create OpenCL input and/or output buffers.\n");
return 1;
}
// 创建内核程序对象
program = clCreateProgramWithSource(context, 1, (const char **)&KernelSource, NULL, &errNum);
if(program == NULL)
{
printf("Failed to create OpenCL program object.\n");
return 1;
}
// 编译内核程序
errNum = clBuildProgram(program, 0, NULL, NULL, NULL, NULL);
if(errNum != CL_SUCCESS)
{
char buildLog[16384];
clGetProgramBuildInfo(program, deviceIDs[0], CL_PROGRAM_BUILD_LOG, sizeof(buildLog), buildLog, NULL);
printf("Error in kernel: %s\n", buildLog);
clReleaseProgram(program);
clReleaseMemObject(input);
clReleaseMemObject(output);
clReleaseCommandQueue(commandQueue);
clReleaseContext(context);
return 1;
}
// 创建内核对象
kernel = clCreateKernel(program, "square", NULL);
if(kernel == NULL)
{
printf("Failed to create OpenCL kernel object.\n");
clReleaseProgram(program);
clReleaseMemObject(input);
clReleaseMemObject(output);
clReleaseCommandQueue(commandQueue);
clReleaseContext(context);
return 1;
}
// 设置内核参数
errNum = clSetKernelArg(kernel, 0, sizeof(cl_mem), &input);
errNum |= clSetKernelArg(kernel, 1, sizeof(cl_mem), &output);
errNum |= clSetKernelArg(kernel, 2, sizeof(unsigned int), &DATA_SIZE);
if(errNum != CL_SUCCESS)
{
printf("Error setting kernel arguments.\n");
clReleaseKernel(kernel);
clReleaseProgram(program);
clReleaseMemObject(input);
clReleaseMemObject(output);
clReleaseCommandQueue(commandQueue);
clReleaseContext(context);
return 1;
}
// 执行内核
size_t globalWorkSize[1] = { DATA_SIZE };
size_t localWorkSize[1] = { 1 };
errNum = clEnqueueNDRangeKernel(commandQueue, kernel, 1, NULL, globalWorkSize, localWorkSize, 0, NULL, NULL);
if(errNum != CL_SUCCESS)
{
printf("Error queuing kernel for execution.\n");
clReleaseKernel(kernel);
clReleaseProgram(program);
clReleaseMemObject(input);
clReleaseMemObject(output);
clReleaseCommandQueue(commandQueue);
clReleaseContext(context);
return 1;
}
// 读取输出缓冲区数据
errNum = clEnqueueReadBuffer(commandQueue, output, CL_TRUE, 0, sizeof(float) * DATA_SIZE, results, 0, NULL, NULL);
if(errNum != CL_SUCCESS)
{
printf("Error reading output buffer.\n");
clReleaseKernel(kernel);
clReleaseProgram(program);
clReleaseMemObject(input);
clReleaseMemObject(output);
clReleaseCommandQueue(commandQueue);
clReleaseContext(context);
return 1;
}
// 验证结果
correct = 0;
for(int i = 0; i < DATA_SIZE; i++)
{
if(results[i] == inputData[i] * inputData[i])
correct++;
}
printf("Computed '%d/%d' correct values!\n", correct, DATA_SIZE);
// 释放资源
clReleaseKernel(kernel);
clReleaseProgram(program);
clReleaseMemObject(input);
clReleaseMemObject(output);
clReleaseCommandQueue(commandQueue);
clReleaseContext(context);
return 0;
}
```
注意,此程序仅是一个示例,实际使用时需要根据具体的 XDMA 硬件和驱动程序进行修改。
相关推荐
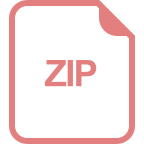
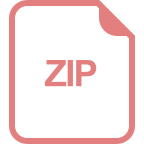
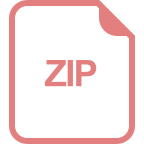














