使用spring-integration-webflux的dsl实现客户端和服务端流式交互
时间: 2024-05-03 11:23:29 浏览: 184
使用spring-integration-webflux的DSL实现客户端和服务端流式交互需要以下步骤:
1. 引入依赖
在pom.xml文件中引入以下依赖:
```xml
<dependency>
<groupId>org.springframework.integration</groupId>
<artifactId>spring-integration-webflux</artifactId>
</dependency>
```
2. 创建服务端
使用WebFlux.fn来创建服务端,示例代码如下:
```java
import org.springframework.http.MediaType;
import org.springframework.integration.dsl.IntegrationFlow;
import org.springframework.integration.dsl.MessageChannels;
import org.springframework.integration.webflux.dsl.WebFlux;
import org.springframework.stereotype.Component;
@Component
public class Server {
public IntegrationFlow serverFlow() {
return IntegrationFlows.from(WebFlux.inboundGateway("/stream")
.requestMapping(m -> m.produces(MediaType.APPLICATION_STREAM_JSON_VALUE))
.requestPayloadType(String.class))
.channel(MessageChannels.flux())
.log()
.map(String::toUpperCase)
.map(s -> s + "-stream")
.get();
}
}
```
该方法创建了一个接收客户端请求的WebFlux.inboundGateway,并将请求传递给MessageChannels.flux()通道,接着执行一些转换操作,并返回结果。在示例中,将请求转换为大写并添加后缀“-stream”。
3. 创建客户端
使用WebFlux.fn来创建客户端,示例代码如下:
```java
import org.springframework.http.MediaType;
import org.springframework.integration.dsl.IntegrationFlow;
import org.springframework.integration.dsl.MessageChannels;
import org.springframework.integration.webflux.dsl.WebFlux;
import org.springframework.stereotype.Component;
@Component
public class Client {
public IntegrationFlow clientFlow() {
return IntegrationFlows.from(MessageChannels.flux())
.handle(WebFlux.outboundGateway("http://localhost:8080/stream")
.httpMethod(HttpMethod.POST)
.expectedResponseType(String.class)
.expectedResponseType(MediaType.APPLICATION_STREAM_JSON_VALUE))
.log()
.get();
}
}
```
该方法从MessageChannels.flux()通道接收请求,并将请求发送到WebFlux.outboundGateway,该网关将请求发送到服务端的“/stream”端点。示例中,期望响应为String类型,MediaType为APPLICATION_STREAM_JSON_VALUE。
4. 创建Spring应用程序
在Spring Boot应用程序的主类中,创建Spring Integration流:
```java
import org.springframework.boot.SpringApplication;
import org.springframework.boot.autoconfigure.SpringBootApplication;
import org.springframework.context.annotation.Bean;
import org.springframework.integration.dsl.IntegrationFlow;
@SpringBootApplication
public class Application {
public static void main(String[] args) {
SpringApplication.run(Application.class, args);
}
@Bean
public IntegrationFlow serverFlow(Server server) {
return server.serverFlow();
}
@Bean
public IntegrationFlow clientFlow(Client client) {
return client.clientFlow();
}
}
```
5. 测试
启动应用程序并访问http://localhost:8080/stream,应该能看到类似以下的输出:
```
2019-12-03 16:37:45.947 INFO 6282 --- [ctor-http-nio-2] o.s.integration.handler.LoggingHandler : GenericMessage [payload=Hello, world!, headers={id=0c9be9f7-191f-8b1a-3f92-12d2c2bd8eaa, contentType=application/json;charset=UTF-8}]
2019-12-03 16:37:45.947 INFO 6282 --- [ctor-http-nio-2] o.s.integration.handler.LoggingHandler : GenericMessage [payload=HELLO, WORLD!-STREAM, headers={id=dcf0d3c6-9bca-2c39-0f7c-5d5b5db5c5a8, contentType=application/json;charset=UTF-8}]
```
这表示客户端已成功将请求发送到服务端,并且服务端已成功执行转换操作并将响应发送回客户端。
阅读全文
相关推荐
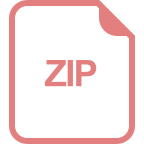
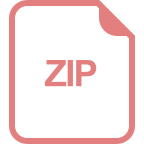
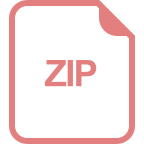















