newList = list.stream().map(item -> { Object object = new Object(); BeanUtils.copyProperties(item , object); return object; }).collect(Collectors.toList()); java还有其他简洁的实现方式吗
时间: 2023-04-03 22:01:28 浏览: 161
可以使用Lombok的@Builder注解来简化代码,如下所示:
@Builder
public class Object {
private String property1;
private int property2;
}
List<Object> newList = list.stream().map(item -> Object.builder()
.property1(item.getProperty1())
.property2(item.getProperty2())
.build())
.collect(Collectors.toList());
相关问题
CivilServiceRequestVO civilServiceRequestVO = new CivilServiceRequestVO(); civilServiceRequestVOList.stream().forEach(item->{ BeanUtils.copyProperties(item,civilServiceRequestVO); } 和 civilServiceRequestVOList.stream().forEach(item->{ CivilServiceRequestVO civilServiceRequestVO = new CivilServiceRequestVO(); BeanUtils.copyProperties(item,civilServiceRequestVO); } 的区别
这两段代码的主要区别在于变量的作用范围和生命周期。
第一段代码中,CivilServiceRequestVO civilServiceRequestVO 是在外部定义的变量,它的作用范围可以是整个方法或者类,而且它的生命周期是整个方法或类的生命周期。在 lambda 表达式中使用它时,每次迭代都会重用这个变量,因此在循环结束后,civilServiceRequestVO 变量将保存最后一次赋值产生的值。
而第二段代码中,CivilServiceRequestVO civilServiceRequestVO 是在 lambda 表达式中定义的局部变量,它的作用范围仅限于 lambda 表达式内部,而且它的生命周期也仅限于 lambda 表达式的执行过程。在 lambda 表达式中使用它时,每次迭代都会创建一个新的变量,因此在循环结束后,civilServiceRequestVO 变量将被销毁。
因此,第一段代码中的 civilServiceRequestVO 变量可以在 lambda 表达式外部继续使用,而第二段代码中的 civilServiceRequestVO 变量只能在 lambda 表达式内部使用。
List<DishDto> dishDtoList =list.stream().map((item) -> { DishDto dishDto = new DishDto(); BeanUtils.copyProperties(item, dishDto); Long categoryId = item.getCategoryId();//分类id //根据id查询分类对象 Category category = categoryService.getById(categoryId); if (category != null) { String categoryName = category.getName(); dishDto.setCategoryName(categoryName); } Long dishId = item.getId();//当前菜品的id LambdaQueryWrapper<DishFlavor> lambdaQueryWrapper = new LambdaQueryWrapper<>(); lambdaQueryWrapper.eq(DishFlavor::getDishId,dishId); List<DishFlavor> dishFlavorList = dishFlavorService.list(lambdaQueryWrapper); dishDto.setFlavors(dishFlavorList); return dishDto; }).collect(Collectors.toList());
这段代码使用了 Java 8 中的 Stream API 对传入的菜品列表 list 进行遍历,并根据每个菜品构建一个 DishDto 对象,最终将所有 DishDto 对象收集到一个列表中。
具体来说,代码中的 map() 方法接收一个 Function 对象,用于将 list 中的每个菜品对象转化为一个 DishDto 对象。在转化的过程中,使用 BeanUtils.copyProperties() 方法将菜品对象的属性值复制到 DishDto 对象中。
接着,代码查询了当前菜品的分类信息和口味信息,并将它们设置到 DishDto 对象中。具体来说:
- 获取当前菜品的分类 ID,根据该 ID 查询分类对象 Category;
- 如果查询到了分类对象,将分类名称设置到 DishDto 对象中;
- 获取当前菜品的 ID,根据该 ID 查询所有关联的口味对象 DishFlavor;
- 将查询到的口味列表设置到 DishDto 对象中。
最终,使用 collect() 方法将所有 DishDto 对象收集到一个列表中,并将该列表作为方法的返回值。
阅读全文
相关推荐
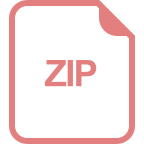
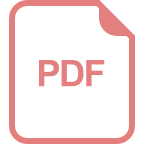
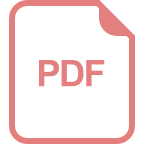














