openCV实现图像的轮廓检测以及外接矩形
时间: 2024-06-08 09:12:29 浏览: 191
要实现图像的轮廓检测以及外接矩形,需要使用OpenCV中的cv2模块。下面是实现的步骤:
1. 读取图像并将其转换为灰度图像。
```python
import cv2
img = cv2.imread('img.jpg')
gray = cv2.cvtColor(img, cv2.COLOR_BGR2GRAY)
```
2. 进行图像的边缘检测。
```python
edges = cv2.Canny(gray, 50, 150)
```
3. 查找图像的轮廓。
```python
contours, _ = cv2.findContours(edges, cv2.RETR_EXTERNAL, cv2.CHAIN_APPROX_SIMPLE)
```
4. 绘制图像的轮廓。
```python
cv2.drawContours(img, contours, -1, (0, 255, 0), 3)
```
5. 查找轮廓的外接矩形。
```python
for contour in contours:
x, y, w, h = cv2.boundingRect(contour)
cv2.rectangle(img, (x, y), (x + w, y + h), (0, 0, 255), 2)
```
完整代码:
```python
import cv2
img = cv2.imread('img.jpg')
gray = cv2.cvtColor(img, cv2.COLOR_BGR2GRAY)
edges = cv2.Canny(gray, 50, 150)
contours, _ = cv2.findContours(edges, cv2.RETR_EXTERNAL, cv2.CHAIN_APPROX_SIMPLE)
cv2.drawContours(img, contours, -1, (0, 255, 0), 3)
for contour in contours:
x, y, w, h = cv2.boundingRect(contour)
cv2.rectangle(img, (x, y), (x + w, y + h), (0, 0, 255), 2)
cv2.imshow('Contours', img)
cv2.waitKey(0)
cv2.destroyAllWindows()
```
阅读全文
相关推荐
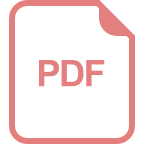
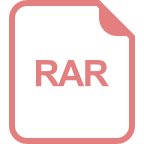
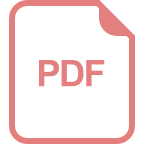















