堆叠自编码器代码python
时间: 2023-11-08 15:58:13 浏览: 38
以下是一个简单的堆叠自编码器的Python代码示例:
```python
import numpy as np
import matplotlib.pyplot as plt
from keras.layers import Input, Dense
from keras.models import Model
# 加载数据集
from keras.datasets import mnist
(x_train, _), (x_test, _) = mnist.load_data()
x_train = x_train.astype('float32') / 255.
x_test = x_test.astype('float32') / 255.
x_train = x_train.reshape((len(x_train), np.prod(x_train.shape[1:])))
x_test = x_test.reshape((len(x_test), np.prod(x_test.shape[1:])))
# 定义输入层
input_layer = Input(shape=(784,))
# 第一层编码器
encoded_layer_1 = Dense(256, activation='relu')(input_layer)
# 第二层编码器
encoded_layer_2 = Dense(128, activation='relu')(encoded_layer_1)
# 第三层编码器
encoded_layer_3 = Dense(64, activation='relu')(encoded_layer_2)
# 第一层解码器
decoded_layer_1 = Dense(128, activation='relu')(encoded_layer_3)
# 第二层解码器
decoded_layer_2 = Dense(256, activation='relu')(decoded_layer_1)
# 输出层
output_layer = Dense(784, activation='sigmoid')(decoded_layer_2)
# 定义模型
autoencoder = Model(input_layer, output_layer)
# 编译模型
autoencoder.compile(optimizer='adadelta', loss='binary_crossentropy')
# 训练模型
autoencoder.fit(x_train, x_train,
epochs=50,
batch_size=256,
shuffle=True,
validation_data=(x_test, x_test))
# 预测重构图像
decoded_imgs = autoencoder.predict(x_test)
# 绘制原始图像与重构图像
n = 10 # 只显示10张图像
plt.figure(figsize=(20, 4))
for i in range(n):
# 原始图像
ax = plt.subplot(2, n, i + 1)
plt.imshow(x_test[i].reshape(28, 28))
plt.gray()
ax.get_xaxis().set_visible(False)
ax.get_yaxis().set_visible(False)
# 重构图像
ax = plt.subplot(2, n, i + 1 + n)
plt.imshow(decoded_imgs[i].reshape(28, 28))
plt.gray()
ax.get_xaxis().set_visible(False)
ax.get_yaxis().set_visible(False)
plt.show()
```
在这个例子中,我们使用了MNIST手写数字数据集。我们首先将像素值归一化到0到1之间,然后将每个图像展平为784维向量。接下来,我们定义了一个三层编码器和三层解码器。每个编码器和解码器层都是全连接的Dense层,并使用ReLU激活函数。最后一层解码器使用sigmoid激活函数,以确保输出值在0到1之间。我们使用ADADelta优化器和二元交叉熵损失函数来训练模型。在训练完成后,我们使用测试数据集来预测和绘制原始图像和重构图像的比较。
相关推荐
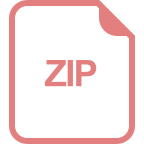
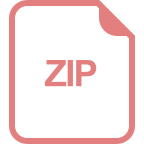














