用堆叠自编码器进行图像分类python代码
时间: 2024-01-07 09:04:34 浏览: 21
以下是使用堆叠自编码器进行图像分类的Python代码:
```python
import tensorflow as tf
import numpy as np
import matplotlib.pyplot as plt
# 加载数据集
(x_train, y_train), (x_test, y_test) = tf.keras.datasets.mnist.load_data()
# 数据预处理
x_train = x_train.astype('float32') / 255.
x_test = x_test.astype('float32') / 255.
x_train = np.reshape(x_train, (len(x_train), 28, 28, 1))
x_test = np.reshape(x_test, (len(x_test), 28, 28, 1))
# 定义自编码器网络结构
input_img = tf.keras.layers.Input(shape=(28, 28, 1))
# 编码层
x = tf.keras.layers.Conv2D(16, (3, 3), activation='relu', padding='same')(input_img)
x = tf.keras.layers.MaxPooling2D((2, 2), padding='same')(x)
x = tf.keras.layers.Conv2D(8, (3, 3), activation='relu', padding='same')(x)
x = tf.keras.layers.MaxPooling2D((2, 2), padding='same')(x)
x = tf.keras.layers.Conv2D(8, (3, 3), activation='relu', padding='same')(x)
encoded = tf.keras.layers.MaxPooling2D((2, 2), padding='same')(x)
# 解码层
x = tf.keras.layers.Conv2D(8, (3, 3), activation='relu', padding='same')(encoded)
x = tf.keras.layers.UpSampling2D((2, 2))(x)
x = tf.keras.layers.Conv2D(8, (3, 3), activation='relu', padding='same')(x)
x = tf.keras.layers.UpSampling2D((2, 2))(x)
x = tf.keras.layers.Conv2D(16, (3, 3), activation='relu')(x)
x = tf.keras.layers.UpSampling2D((2, 2))(x)
decoded = tf.keras.layers.Conv2D(1, (3, 3), activation='sigmoid', padding='same')(x)
# 创建自编码器模型
autoencoder = tf.keras.models.Model(input_img, decoded)
autoencoder.compile(optimizer='adadelta', loss='binary_crossentropy')
# 训练自编码器
autoencoder.fit(x_train, x_train, epochs=50, batch_size=128, shuffle=True, validation_data=(x_test, x_test))
# 创建分类器模型
encoded_input = tf.keras.layers.Input(shape=(4, 4, 8))
x = tf.keras.layers.Flatten()(encoded_input)
x = tf.keras.layers.Dense(64, activation='relu')(x)
output = tf.keras.layers.Dense(10, activation='softmax')(x)
classifier = tf.keras.models.Model(encoded_input, output)
# 冻结自编码器的权重
for layer in autoencoder.layers:
layer.trainable = False
# 将自编码器的编码层作为分类器的输入
encoded_imgs = tf.keras.layers.Input(shape=(4, 4, 8))
classification = classifier(encoded_imgs)
# 创建整个模型
stacked_model = tf.keras.models.Model(encoded_imgs, classification)
# 编译整个模型
stacked_model.compile(optimizer='rmsprop', loss='categorical_crossentropy', metrics=['accuracy'])
# 训练整个模型
stacked_model.fit(x_train, y_train, epochs=20, batch_size=128, shuffle=True, validation_data=(x_test, y_test))
# 评估模型性能
score = stacked_model.evaluate(x_test, y_test, verbose=0)
print('Test loss:', score[0])
print('Test accuracy:', score[1])
```
这个代码使用了一个堆叠自编码器来进行图像分类。首先,我们定义了一个自编码器网络结构,然后训练自编码器来学习图像的特征表示。接着,我们创建一个分类器模型,使用自编码器的编码层作为输入。然后,我们创建整个模型,将自编码器的编码层作为输入,将分类器作为输出。最后,我们训练整个模型,并评估其性能。
相关推荐
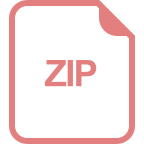
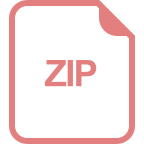
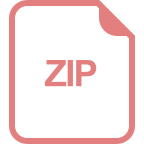














