用java写一个对象数组 (1)输入多个学生的学号,姓名,数学,语文,英语成绩。 (2)按总分从大到小排序并输出。 (3)在main方法中进行测试。
时间: 2024-05-27 16:14:23 浏览: 37
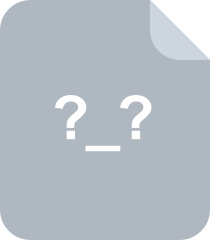
第一个数组内有学号、姓名、第二个数组有《高等数学》、《线性代数》、《Java程序设计》、《大学英语》、每个人的总成绩和平均成
以下是Java代码:
import java.util.Scanner;
public class Student {
private String id;
private String name;
private double mathScore;
private double chineseScore;
private double englishScore;
private double totalScore;
public Student(String id, String name, double mathScore, double chineseScore, double englishScore) {
this.id = id;
this.name = name;
this.mathScore = mathScore;
this.chineseScore = chineseScore;
this.englishScore = englishScore;
this.totalScore = mathScore + chineseScore + englishScore;
}
public String getId() {
return id;
}
public String getName() {
return name;
}
public double getMathScore() {
return mathScore;
}
public double getChineseScore() {
return chineseScore;
}
public double getEnglishScore() {
return englishScore;
}
public double getTotalScore() {
return totalScore;
}
}
public class Main {
public static void main(String[] args) {
Scanner scanner = new Scanner(System.in);
System.out.print("请输入学生人数:");
int n = scanner.nextInt();
Student[] students = new Student[n];
for (int i = 0; i < n; i++) {
System.out.print("请输入第" + (i + 1) + "个学生的学号:");
String id = scanner.next();
System.out.print("请输入第" + (i + 1) + "个学生的姓名:");
String name = scanner.next();
System.out.print("请输入第" + (i + 1) + "个学生的数学成绩:");
double mathScore = scanner.nextDouble();
System.out.print("请输入第" + (i + 1) + "个学生的语文成绩:");
double chineseScore = scanner.nextDouble();
System.out.print("请输入第" + (i + 1) + "个学生的英语成绩:");
double englishScore = scanner.nextDouble();
students[i] = new Student(id, name, mathScore, chineseScore, englishScore);
}
for (int i = 0; i < n - 1; i++) {
for (int j = i + 1; j < n; j++) {
if (students[i].getTotalScore() < students[j].getTotalScore()) {
Student temp = students[i];
students[i] = students[j];
students[j] = temp;
}
}
}
System.out.println("按总分从大到小排序后的学生信息如下:");
for (int i = 0; i < n; i++) {
System.out.println("学号:" + students[i].getId() + ",姓名:" + students[i].getName() +
",数学成绩:" + students[i].getMathScore() + ",语文成绩:" + students[i].getChineseScore() +
",英语成绩:" + students[i].getEnglishScore() + ",总分:" + students[i].getTotalScore());
}
}
}
阅读全文
相关推荐
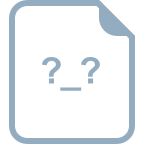
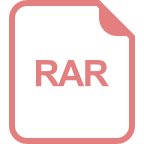




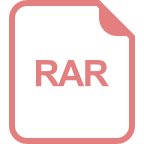

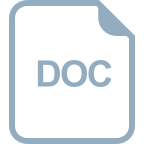
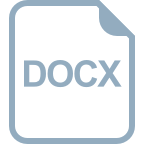
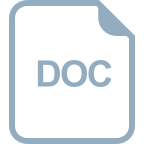
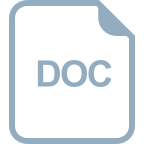
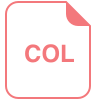


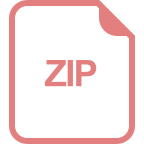
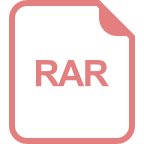