01背包问题蚁群算法
时间: 2024-05-10 07:11:59 浏览: 21
01背包问题是一个经典的组合优化问题,蚁群算法是一种基于模拟蚂蚁觅食行为的启发式算法。蚁群算法通过模拟蚂蚁在搜索空间中的移动和信息素的更新来寻找问题的最优解。
在01背包问题中,给定一组物品,每个物品有一个重量和一个价值,同时给定一个背包的容量。目标是选择一些物品放入背包中,使得放入背包的物品总重量不超过背包容量,同时总价值最大化。
蚁群算法解决01背包问题的思路是将每个物品看作一个蚂蚁,每个蚂蚁根据一定的规则选择是否携带该物品。蚂蚁在搜索过程中会根据当前的信息素浓度和启发式信息进行决策。信息素浓度表示了当前路径的好坏程度,启发式信息表示了物品的重要性。
具体来说,蚁群算法的步骤如下:
1. 初始化一群蚂蚁,并随机分配物品给每只蚂蚁。
2. 每只蚂蚁根据一定的规则选择是否携带物品,并计算当前携带物品的总价值。
3. 更新信息素浓度,根据蚂蚁的选择结果更新信息素浓度。
4. 重复步骤2和步骤3,直到达到停止条件(例如达到最大迭代次数)。
5. 选择价值最高的解作为最优解。
蚁群算法通过模拟蚂蚁的行为和信息素的更新,能够在搜索空间中找到较好的解。它具有全局搜索能力和自适应性,适用于求解组合优化问题。
相关问题
01背包问题蚁群算法python
以下是使用蚁群算法解决01背包问题的Python代码:
```python
import random
# 蚂蚁数量
ant_count = 50
# 迭代次数
iterations = 200
# 信息素挥发系数
rho = 0.1
# 信息素强度
Q = 1
# 信息素启发因子
alpha = 1
# 距离启发因子
beta = 2
# 最大质量
max_weight = 100
# 物品数量
item_count = 20
# 物品重量
item_weights = [random.randint(1, 10) for _ in range(item_count)]
# 物品价值
item_values = [random.randint(1, 10) for _ in range(item_count)]
# 信息素矩阵
pheromone = [[1.0 for _ in range(max_weight + 1)] for _ in range(item_count)]
# 最优解
best_solution = []
best_value = 0
# 计算每个物品的价值密度
def calculate_density():
density = []
for i in range(item_count):
density.append(item_values[i] / item_weights[i])
return density
# 计算每个物品被选中的概率
def calculate_probabilities(ant, selected_items, density):
probabilities = []
total_prob = 0
for i in range(item_count):
if i not in selected_items:
if ant['current_weight'] + item_weights[i] <= max_weight:
p = pow(pheromone[i][ant['current_weight'] + item_weights[i]], alpha) * pow(density[i], beta)
probabilities.append(p)
total_prob += p
else:
probabilities.append(0)
else:
probabilities.append(0)
if total_prob == 0:
return [1 / (item_count - len(selected_items)) if i not in selected_items else 0 for i in range(item_count)]
else:
return [p / total_prob if i not in selected_items else 0 for i, p in enumerate(probabilities)]
# 选择下一个物品
def select_next_item(ant, selected_items, density):
probabilities = calculate_probabilities(ant, selected_items, density)
r = random.uniform(0, 1)
total_prob = 0
for i, p in enumerate(probabilities):
total_prob += p
if total_prob >= r:
return i
return -1
# 更新信息素矩阵
def update_pheromone():
global pheromone
for i in range(item_count):
for j in range(max_weight + 1):
pheromone[i][j] *= (1 - rho)
for ant in ants:
if ant['value'] > 0:
pheromone[i][j] += (Q / ant['value']) * ant['items'].count(i) * int(j == ant['current_weight'])
# 初始化蚂蚁
def init_ants():
ants = []
for i in range(ant_count):
ant = {'items': [], 'value': 0, 'current_weight': 0}
ants.append(ant)
return ants
# 计算蚂蚁的价值和重量
def calculate_ant_value(ant):
value = 0
weight = 0
for i in ant['items']:
value += item_values[i]
weight += item_weights[i]
ant['value'] = value
ant['current_weight'] = weight
# 蚁群算法主函数
def ant_colony_optimization():
global best_solution, best_value
density = calculate_density()
ants = init_ants()
for it in range(iterations):
for ant in ants:
selected_items = []
while ant['current_weight'] < max_weight:
next_item = select_next_item(ant, selected_items, density)
if next_item == -1:
break
ant['items'].append(next_item)
selected_items.append(next_item)
ant['current_weight'] += item_weights[next_item]
calculate_ant_value(ant)
if ant['value'] > best_value:
best_solution = ant['items']
best_value = ant['value']
update_pheromone()
return best_solution, best_value
# 测试
best_solution, best_value = ant_colony_optimization()
print('最优解:', best_solution)
print('最优价值:', best_value)
```
背包问题蚁群算法matlab
背包问题是一个经典的组合优化问题,其目标是在给定的背包容量下选择一组物品,使得物品的总价值最大化,同时保证总重量不超过背包的容量。蚁群算法是一种基于群体智能的优化算法,它通过模拟蚂蚁在寻找食物过程中的行为来解决问题。
在使用蚁群算法解决背包问题时,首先需要将每个物品编码为一个二进制字符串,其中每一位表示是否选择该物品放入背包。然后,根据蚂蚁的行为规则,使用概率选择策略确定每个蚂蚁在每一步中选择哪个物品放入背包。
接下来,根据蚁群算法的迭代过程,在每一次迭代中,根据蚂蚁的选择结果计算出每个蚂蚁的适应度值,并根据适应度值更新信息素矩阵。信息素矩阵代表了蚂蚁在搜索过程中的行为路径,通过信息素的更新和挥发,蚂蚁能够逐渐找到最优解。
最后,通过多次迭代,蚁群算法可以找到近似最优的背包解决方案。在MATLAB中,可以使用蚁群算法的相关工具包或自行编写代码来实现。
相关推荐
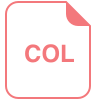











