c++queue在函数之间传递
时间: 2023-08-03 14:25:44 浏览: 580
可以使用引用或指针来在函数之间传递C++ STL队列。如果要传递队列作为函数参数,可以使用引用参数或指针参数。以下是一个使用引用参数传递队列的示例代码:
```c++
#include <iostream>
#include <queue>
using namespace std;
void processQueue(queue<int>& q) {
while (!q.empty()) {
int val = q.front();
cout << val << " ";
q.pop();
}
cout << endl;
}
int main() {
queue<int> q;
q.push(10);
q.push(20);
q.push(30);
processQueue(q);
return 0;
}
```
在这个示例中,我们定义了一个名为`processQueue`的函数,它的参数是一个引用类型的队列。在`main`函数中,我们创建了一个队列`q`,然后将三个整数值10、20和30添加到队列中。接下来,我们将这个队列作为参数传递给`processQueue`函数,该函数将打印出队列中的所有元素并将其弹出。
相关问题
c++定义priority_queue的比较函数
在 C++ 中,可以使用 `std::priority_queue` 来定义优先队列。要定义比较函数,你可以使用函数指针、函数对象或者 Lambda 表达式。下面是几种不同的方法:
1. 使用函数指针:
```cpp
bool compare(int a, int b) {
// 自定义的比较逻辑,返回 true 表示 a 在 b 之前
// 返回 false 表示 a 在 b 之后
// 例如,按照升序排列:
return a > b;
}
std::priority_queue<int, std::vector<int>, bool (*)(int, int)> pq(compare);
```
2. 使用函数对象(重载 `operator()`):
```cpp
struct Compare {
bool operator()(int a, int b) {
// 自定义的比较逻辑,返回 true 表示 a 在 b 之前
// 返回 false 表示 a 在 b 之后
// 例如,按照升序排列:
return a > b;
}
};
std::priority_queue<int, std::vector<int>, Compare> pq;
```
3. 使用 Lambda 表达式:
```cpp
auto compare = [](int a, int b) {
// 自定义的比较逻辑,返回 true 表示 a 在 b 之前
// 返回 false 表示 a 在 b 之后
// 例如,按照升序排列:
return a > b;
};
std::priority_queue<int, std::vector<int>, decltype(compare)> pq(compare);
```
无论使用哪种方法,都需要确保比较函数能够正确判断元素的优先级。在创建 `std::priority_queue` 对象时,将比较函数作为第三个模板参数传递给它。
c++队列优先queue使用和成员函数
C++标准库中的`std::priority_queue`是一个基于堆的数据结构,它实现了优先队列的概念,即新插入的元素默认会被放置在队列顶部,保证了每次出队的是优先级最高的元素。以下是`priority_queue`的基本使用和一些重要成员函数:
1. **创建**:
```cpp
#include <queue>
std::priority_queue<int> pq; // 默认采用最大堆(大根堆),可以传递比较函数自定义排序
```
如果你想要最小堆(小根堆),可以传递`std::greater<int>`作为第三个模板参数:
```cpp
std::priority_queue<int, std::vector<int>, std::less<int>> minPQ;
```
2. **插入元素**:
```cpp
pq.push(5);
pq.push(2);
```
3. **获取并删除最高优先级元素**(顶元素):
```cpp
int topElement = pq.top(); // 获取当前优先级最高的元素
pq.pop(); // 删除并返回顶元素
```
4. **查看元素大小**:
```cpp
bool empty() const { return pq.empty(); } // 判断是否为空
size_t size() const { return pq.size(); } // 返回元素数量
```
5. **修改优先级**:
由于`priority_queue`是自动维护堆性质的,你不能直接改变元素的优先级。如果需要动态调整,你可能需要先删除元素,然后用新的优先级重新插入。
6. **成员函数举例**:
```cpp
// 示例代码
while (!pq.empty()) {
int currentPriority = pq.top();
pq.pop();
process(currentPriority); // 按优先级处理元素
}
```
阅读全文
相关推荐
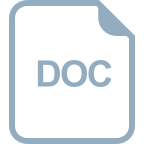
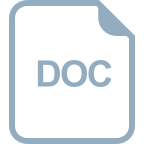
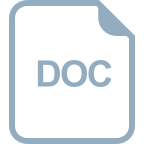
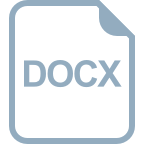
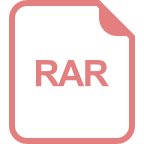
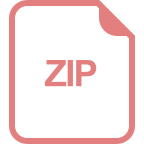
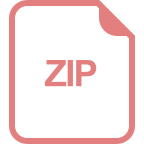
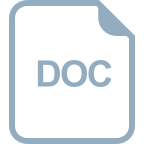
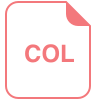







