如何用c语言创建单链表
时间: 2023-08-06 11:07:03 浏览: 43
要使用 C 语言创建单链表,可以按照以下步骤进行:
1. 定义一个结构体,用于表示链表的节点,包括一个数据域和一个指向下一个节点的指针域,例如:
```
struct ListNode {
int val;
struct ListNode *next;
};
```
2. 创建链表的头节点,即一个空节点,通常命名为 `head`,并让其指向 NULL,例如:
```
struct ListNode *head = NULL;
```
3. 插入一个节点到链表中,可以先创建一个新的节点,然后将其插入到链表的合适位置。例如,在链表末尾插入一个节点可以使用以下代码:
```
struct ListNode *newNode = (struct ListNode*) malloc(sizeof(struct ListNode));
newNode->val = value;
newNode->next = NULL;
if (head == NULL) {
head = newNode;
} else {
struct ListNode *cur = head;
while (cur->next != NULL) {
cur = cur->next;
}
cur->next = newNode;
}
```
其中,`value` 表示新节点的值。
4. 遍历链表,可以使用以下代码:
```
struct ListNode *cur = head;
while (cur != NULL) {
printf("%d ", cur->val);
cur = cur->next;
}
```
其中,`printf` 函数用于输出节点的值。
5. 最后,别忘了释放链表节点的内存,可以使用以下代码:
```
struct ListNode *cur = head;
while (cur != NULL) {
struct ListNode *next = cur->next;
free(cur);
cur = next;
}
head = NULL;
```
相关推荐
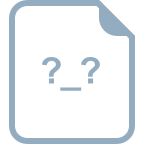
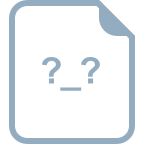














