def build_model(max_features=1, maxlen=65): """Build LSTM model""" model = Sequential() model.add(Embedding(max_features, 128, input_length=maxlen)) model.add(LSTM(128)) model.add(Dropout(0.5)) model.add(Dense(1)) model.add(Activation('sigmoid')) # model.add(tf.keras.layers.BatchNormalization()) model.compile(loss='binary_crossentropy', optimizer='rmsprop') return model def data(Domain): valid_chars = pickle.load(open('dictionary.plk','rb')) Domain = [[valid_chars[y] for y in x] for x in Domain] Domain = sequence.pad_sequences(Domain, maxlen=65) return Domain def run(Domain): Domains = data(Domain) modelaaa = build_model(max_features=1, maxlen=65) modelaaa.load_weights('Mymodel.h5') predictions = model.predict(Domains) return predictions if __name__ == "__main__": print(run(['baidu.com'])) # Run with 1 to make it fast报错Unable to load weights saved in HDF5 format into a subclassed Model which has not created its variables yet. Call the Model first, then load the weights.怎么改
时间: 2024-03-07 15:49:46 浏览: 62
在 `run` 函数中,我们应该先创建模型,再加载权重。所以我们需要将创建模型和加载权重的顺序调换一下,同时将模型命名为 `modelaaa`,但在 `predict` 中使用的却是 `model`,需要将其改为 `modelaaa`。修改后的代码如下:
```
def run(Domain):
Domains = data(Domain)
modelaaa = build_model(max_features=1, maxlen=65)
modelaaa.load_weights('Mymodel.h5')
predictions = modelaaa.predict(Domains)
return predictions
```
这样就可以避免 `Unable to load weights saved in HDF5 format into a subclassed Model which has not created its variables yet. Call the Model first, then load the weights.` 的错误了。
相关问题
import numpy as np from keras.preprocessing import sequence from keras_preprocessing import sequence from keras.models import Sequential from keras.layers.core import Dense, Dropout, Activation from tensorflow.python.keras.layers.embeddings import Embedding from keras.layers import LSTM import sklearn import pickle # from sklearn.cross_validation import train_test_split import sklearn.model_selection from sklearn.model_selection import train_test_split from keras.models import load_model def build_model(max_features=1, maxlen=65): """Build LSTM model""" model = Sequential() model.add(Embedding(max_features, 128, input_length=maxlen)) model.add(LSTM(128)) model.add(Dropout(0.5)) model.add(Dense(1)) model.add(Activation('sigmoid')) # model.add(tf.keras.layers.BatchNormalization()) model.compile(loss='binary_crossentropy', optimizer='rmsprop') return model def data(Domain): valid_chars = pickle.load(open('dictionary.plk','rb')) Domain = [[valid_chars[y] for y in x] for x in Domain] Domain = sequence.pad_sequences(Domain, maxlen=65) return Domain def run(Domain): Domains=data(Domain) model = build_model() model.load_weights('Mymodel.h5') predictions = model.predict(Domains) return predictions if name == "main": print(run(['baidu.com'])) # Run with 1 to make it fast
这段代码是一个使用 LSTM 模型对域名进行分类的程序,其中包括了模型的构建和加载,以及对数据进行预处理和预测。具体来说,该程序使用了 Keras 和 TensorFlow 库进行模型的建立和训练,同时使用了 pickle 库进行数据的读取和存储。在运行该程序时,输入一个域名进行分类,输出该域名属于恶意或者良性的概率。
def build_model(max_features, maxlen): """Build LSTM model""" model = Sequential() model.add(Embedding(max_features, 128, input_length=maxlen)) model.add(LSTM(128)) model.add(Dropout(0.5)) model.add(Dense(1)) model.add(Activation('sigmoid')) # model.add(tf.keras.layers.BatchNormalization()) model.compile(loss='binary_crossentropy', optimizer='rmsprop') return model怎么model.build
这个函数中的模型是使用Keras的Sequential API构建的,而不是使用Functional API或Subclassing API。在这种情况下,我们不需要使用“model.build”方法来构建模型,因为它已经在“Sequential”类中自动完成了。
“Sequential”类是Keras中最简单的模型容器,它按顺序将各个网络层堆叠在一起,从而形成一个完整的神经网络模型。在这个函数中,我们首先使用“model.add”方法来添加一个嵌入层(Embedding)、LSTM层(LSTM)、Dropout层(Dropout)和一个密集层(Dense)到模型中,然后在最后添加一个sigmoid激活函数(Activation)来输出二元分类结果。
在这个函数中,我们已经定义好了模型的结构和参数,并使用“model.compile”方法来编译模型。这个方法接受损失函数(loss)、优化器(optimizer)和评估指标(metrics)等参数,然后构建出一个可训练的模型。最后,我们将这个模型返回给调用者。
阅读全文
相关推荐
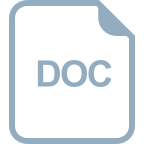
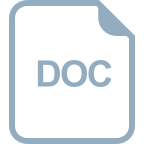
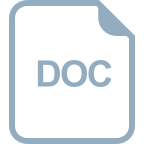





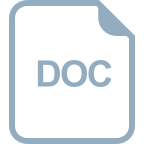
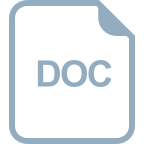
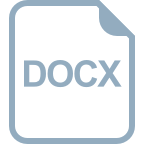
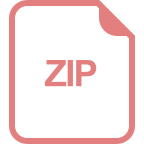