设计一个整数顺序表,包含了基本运算程序(插入,删除,求长度等等),并用相关数据测试
时间: 2024-09-22 21:10:02 浏览: 56
设计一个整数顺序表(也称为线性表或数组列表)可以使用数组作为底层存储结构,并提供一些基本的操作方法,如插入、删除和查询长度。这里是一个简单的Python实现:
```python
class IntegerList:
def __init__(self):
self.data = []
# 插入元素
def insert(self, index, value):
if index < 0 or index > len(self.data):
raise IndexError("Index out of range")
self.data.insert(index, value)
# 删除元素
def remove(self, index):
if index < 0 or index >= len(self.data):
raise IndexError("Index out of range")
del self.data[index]
# 求长度
def length(self):
return len(self.data)
# 测试用例
def test(self):
list = IntegerList()
list.insert(0, 5)
list.insert(1, 3)
list.insert(2, 7)
assert list.length() == 3
print(f"Length before delete: {list.length()}")
list.remove(1)
print(f"Length after delete: {list.length()}")
try:
list.remove(4) # 非法索引,会抛出异常
except IndexError as e:
print(e)
# 使用测试数据
test_list = IntegerList()
test_list.test()
```
在这个例子中,`IntegerList`类包含三个核心操作:`insert`用于在指定位置插入元素,`remove`用于删除指定位置的元素,`length`返回列表的当前长度。`test`方法展示了如何使用这些函数以及处理异常。
阅读全文
相关推荐
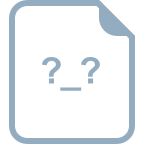
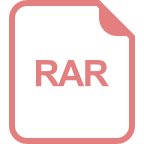
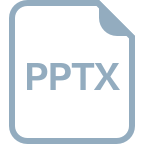


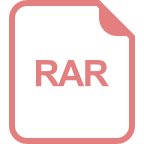
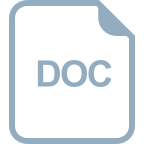
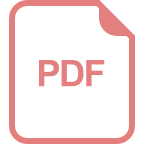
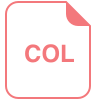
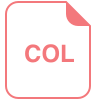
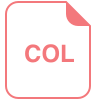
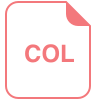
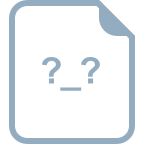
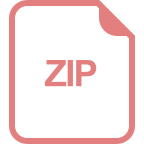
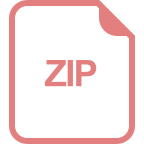
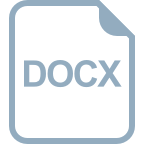