爬取天气数据并可视化的代码
时间: 2023-09-05 14:15:23 浏览: 203
以下是一个使用Python爬取天气数据并进行可视化的示例代码:
```python
import requests
import matplotlib.pyplot as plt
# 爬取天气数据
def get_weather_data(city):
url = f"http://api.weatherapi.com/v1/current.json?key=YOUR_API_KEY&q={city}"
response = requests.get(url)
data = response.json()
return data
# 解析天气数据
def parse_weather_data(data):
temperature = data["current"]["temp_c"]
humidity = data["current"]["humidity"]
wind_speed = data["current"]["wind_kph"]
return temperature, humidity, wind_speed
# 可视化天气数据
def visualize_weather_data(city, temperature, humidity, wind_speed):
labels = ["Temperature (°C)", "Humidity (%)", "Wind Speed (km/h)"]
values = [temperature, humidity, wind_speed]
plt.bar(labels, values)
plt.title(f"Weather Information for {city}")
plt.xlabel("Measurements")
plt.ylabel("Values")
plt.show()
# 主程序
def main():
city = input("请输入城市名称:")
weather_data = get_weather_data(city)
if "error" in weather_data:
print(f"获取天气数据失败:{weather_data['error']['message']}")
return
temperature, humidity, wind_speed = parse_weather_data(weather_data)
visualize_weather_data(city, temperature, humidity, wind_speed)
if __name__ == "__main__":
main()
```
请替换`YOUR_API_KEY`为你自己的天气API密钥。这个示例使用了[WeatherAPI](https://www.weatherapi.com/)来获取天气数据,并使用matplotlib库进行可视化。你可以根据自己的需求修改代码,例如使用其他天气API或采用其他可视化工具。
阅读全文
相关推荐
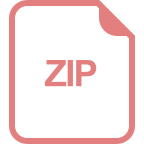

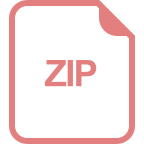
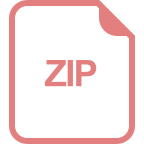
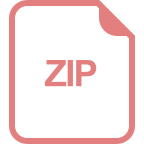
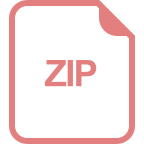
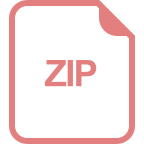
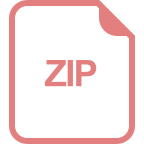
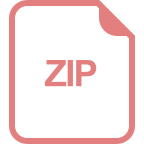
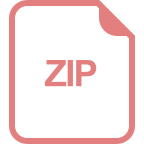
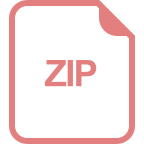




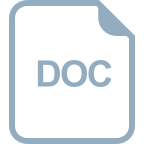
