使用netcdf库读取nc文件的c语言代码
时间: 2023-08-02 15:09:40 浏览: 140
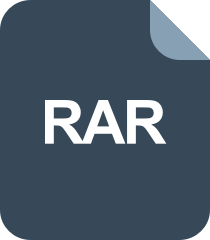
netcdf.rar_Fortran nc_nc fortran_nc文件_netcdf
以下是使用netcdf库读取nc文件的c语言代码示例:
```c
#include <stdio.h>
#include <stdlib.h>
#include <netcdf.h>
#define FILE_NAME "example.nc"
int main()
{
int ncid, varid;
int status;
size_t latlen, lonlen;
float *lat, *lon, *data;
// Open the file
if ((status = nc_open(FILE_NAME, NC_NOWRITE, &ncid)))
goto error;
// Get the variable id
if ((status = nc_inq_varid(ncid, "lat", &varid)))
goto error;
// Get the variable length
if ((status = nc_inq_dimlen(ncid, varid, &latlen)))
goto error;
// Allocate memory for the variable
lat = (float*) malloc(latlen * sizeof(float));
// Read the variable data
if ((status = nc_get_var_float(ncid, varid, lat)))
goto error;
// Repeat for the "lon" variable
if ((status = nc_inq_varid(ncid, "lon", &varid)))
goto error;
if ((status = nc_inq_dimlen(ncid, varid, &lonlen)))
goto error;
lon = (float*) malloc(lonlen * sizeof(float));
if ((status = nc_get_var_float(ncid, varid, lon)))
goto error;
// Repeat for the "data" variable
if ((status = nc_inq_varid(ncid, "data", &varid)))
goto error;
data = (float*) malloc(latlen * lonlen * sizeof(float));
if ((status = nc_get_var_float(ncid, varid, data)))
goto error;
// Output the data
for (size_t i = 0; i < latlen; i++) {
for (size_t j = 0; j < lonlen; j++) {
printf("(%f, %f): %f\n", lat[i], lon[j], data[i*lonlen+j]);
}
}
// Close the file and free the memory
nc_close(ncid);
free(lat);
free(lon);
free(data);
return 0;
error:
printf("Error: %s\n", nc_strerror(status));
return 1;
}
```
这段代码打开名为 "example.nc" 的文件,读取其中名为 "lat"、"lon" 和 "data" 的三个变量,并将它们的值输出到屏幕上。在使用该代码时,请确保在编译时链接 netcdf 库。
阅读全文
相关推荐
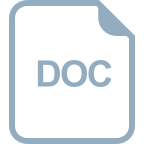
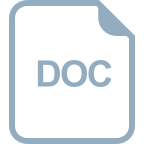

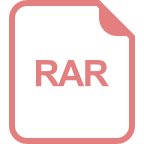
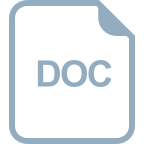
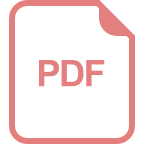
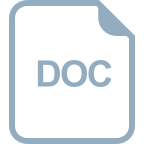
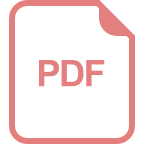
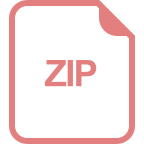
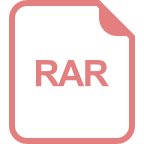
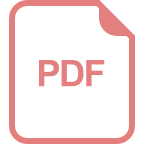
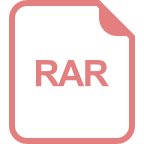
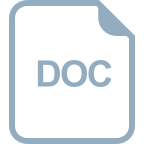
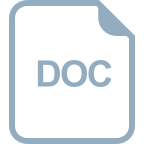
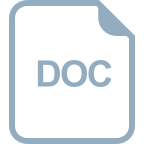
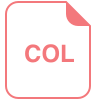
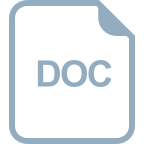
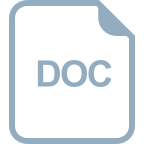
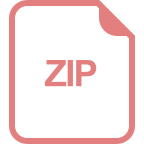