哈希表求模代码并遍历寻找C++
时间: 2024-05-16 14:15:55 浏览: 160
以下是哈希表求模的代码:
```python
def hash_function(key, size):
return key % size
```
其中,`key`是要进行哈希的键值,`size`是哈希表的大小。
接下来是遍历寻找C的代码:
```python
def find_c(hash_table):
for key, value in hash_table.items():
if value == 'C':
return key
return None
```
其中,`hash_table`是一个字典,表示哈希表。该函数会遍历哈希表中所有的键值对,寻找值为'C'的键值对。如果找到了,就返回对应的键值;如果遍历完了哈希表都没有找到,就返回`None`。
相关问题
C++哈希表求模代码并遍历寻找
下面是一个使用哈希表求模的示例代码,它可以插入整数到哈希表中,并通过哈希表遍历函数查找特定的值:
```c
#include <stdio.h>
#include <stdlib.h>
#define TABLE_SIZE 10
typedef struct HashNode {
int data;
struct HashNode* next;
} HashNode;
typedef struct {
HashNode* buckets[TABLE_SIZE];
} HashTable;
int hash(int data) {
return data % TABLE_SIZE;
}
void insert(HashTable* table, int data) {
int index = hash(data);
HashNode* node = (HashNode*)malloc(sizeof(HashNode));
node->data = data;
node->next = table->buckets[index];
table->buckets[index] = node;
}
int find(HashTable* table, int data) {
int index = hash(data);
HashNode* node = table->buckets[index];
while (node != NULL) {
if (node->data == data) {
return 1;
}
node = node->next;
}
return 0;
}
void traverse(HashTable* table) {
for (int i = 0; i < TABLE_SIZE; i++) {
HashNode* node = table->buckets[i];
printf("Bucket %d:", i);
while (node != NULL) {
printf(" %d", node->data);
node = node->next;
}
printf("\n");
}
}
int main() {
HashTable table = { 0 };
insert(&table, 5);
insert(&table, 15);
insert(&table, 25);
insert(&table, 35);
traverse(&table);
printf("Find 15: %d\n", find(&table, 15));
printf("Find 20: %d\n", find(&table, 20));
return 0;
}
```
这个示例代码创建了一个大小为10的哈希表,并使用求模函数作为哈希函数。它可以插入整数到哈希表中,并通过哈希表遍历函数遍历哈希表。最后,它使用查找函数查找特定的值。
C++中哈希表如何遍历
在C++中,哈希表可以使用迭代器或范围循环进行遍历。
使用迭代器遍历哈希表的示例代码如下:
```c++
#include <iostream>
#include <unordered_map>
int main() {
std::unordered_map<std::string, int> myMap = { {"apple", 1}, {"banana", 2}, {"orange", 3} };
// 使用迭代器遍历哈希表
for (auto it = myMap.begin(); it != myMap.end(); ++it) {
std::cout << it->first << ": " << it->second << std::endl;
}
return 0;
}
```
使用范围循环遍历哈希表的示例代码如下:
```c++
#include <iostream>
#include <unordered_map>
int main() {
std::unordered_map<std::string, int> myMap = { {"apple", 1}, {"banana", 2}, {"orange", 3} };
// 使用范围循环遍历哈希表
for (const auto& [key, value] : myMap) {
std::cout << key << ": " << value << std::endl;
}
return 0;
}
```
其中,使用auto关键字定义的迭代器it指向哈希表的第一个元素,每次遍历时先判断迭代器是否等于哈希表的end()迭代器,如果不等于,则输出当前元素的键值对,然后将迭代器指向下一个元素。使用范围循环则更加简洁,直接遍历哈希表的所有元素并输出。
阅读全文
相关推荐
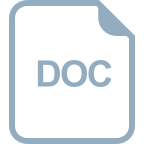
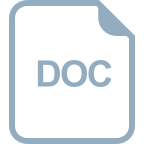
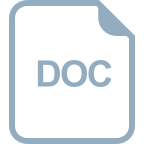

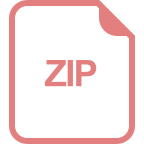
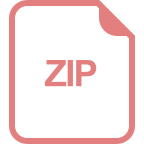
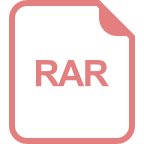
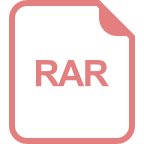
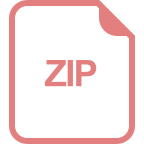
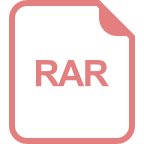
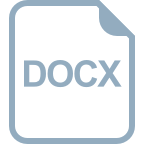
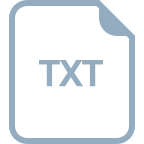
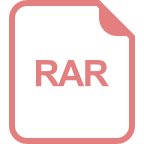
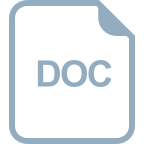


