c++利用多态求各种图形面积坐标Point
时间: 2024-05-06 11:18:24 浏览: 15
首先,我们需要定义一个抽象基类`Shape`,用于表示图形。该类中包含两个纯虚函数:`getArea()`用于计算图形的面积,`printCoordinates()`用于打印图形的坐标。
```c++
class Shape {
public:
virtual double getArea() = 0;
virtual void printCoordinates() = 0;
};
```
然后,我们可以定义各种具体的图形类,例如矩形、圆形等,这些类都继承自`Shape`类。在这些类中,我们需要实现基类中定义的两个纯虚函数,并添加该类特有的属性和方法。
以矩形为例,我们可以定义一个`Rectangle`类,其中包含`width`和`height`两个属性表示矩形的宽和高。
```c++
class Rectangle : public Shape {
private:
int width;
int height;
public:
Rectangle(int w, int h) : width(w), height(h) {}
double getArea() override { return width * height; }
void printCoordinates() override { cout << "Rectangle coordinates: (0, 0), (" << width << ", 0), (" << width << ", " << height << "), (0, " << height << ")" << endl; }
};
```
同样地,我们可以定义一个圆形类`Circle`,其中包含`radius`属性表示圆形的半径。
```c++
class Circle : public Shape {
private:
int radius;
public:
Circle(int r) : radius(r) {}
double getArea() override { return 3.14159 * radius * radius; }
void printCoordinates() override { cout << "Circle coordinates: center(0, 0), radius=" << radius << endl; }
};
```
最后,我们可以在主函数中创建各种图形对象,并通过基类指针调用其成员函数。
```c++
int main() {
Shape* shapes[2];
shapes[0] = new Rectangle(5, 10);
shapes[1] = new Circle(7);
for (int i = 0; i < 2; i++) {
shapes[i]->printCoordinates();
cout << "Area: " << shapes[i]->getArea() << endl;
}
return 0;
}
```
输出结果如下:
```
Rectangle coordinates: (0, 0), (5, 0), (5, 10), (0, 10)
Area: 50
Circle coordinates: center(0, 0), radius=7
Area: 153.937
```
相关推荐
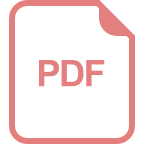
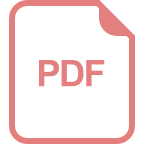
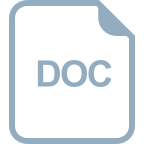














