深度优先求解迷宫问题,输入迷宫数组,输出迷宫横向走标记为L,竖向走标记为H,拐弯标记为W,用C++语言编程
时间: 2024-03-16 20:46:30 浏览: 54
好的,以下是一个基于深度优先搜索的迷宫求解程序,将横向走标记为L,竖向走标记为H,拐弯标记为W。请注意,本程序并没有进行输入检查,使用时请确保输入的迷宫数组符合要求。
```c++
#include <iostream>
#include <vector>
using namespace std;
const int N = 110;
char maze[N][N];
bool visited[N][N];
int n, m;
vector<pair<int, int>> path;
bool dfs(int x, int y, int px, int py, char dir) {
visited[x][y] = true;
path.emplace_back(x, y);
if (x == n && y == m) return true;
if (x + 1 <= n && maze[x + 1][y] == '.' && !visited[x + 1][y]) {
if (dir == 'V') maze[x][y] = 'W';
if (dfs(x + 1, y, x, y, 'V')) return true;
}
if (y + 1 <= m && maze[x][y + 1] == '.' && !visited[x][y + 1]) {
if (dir == 'H') maze[x][y] = 'W';
if (dfs(x, y + 1, x, y, 'H')) return true;
}
if (y - 1 >= 1 && maze[x][y - 1] == '.' && !visited[x][y - 1]) {
if (dir == 'H') maze[x][y] = 'W';
if (dfs(x, y - 1, x, y, 'H')) return true;
}
if (x - 1 >= 1 && maze[x - 1][y] == '.' && !visited[x - 1][y]) {
if (dir == 'V') maze[x][y] = 'W';
if (dfs(x - 1, y, x, y, 'V')) return true;
}
visited[x][y] = false;
path.pop_back();
return false;
}
int main() {
cin >> n >> m;
for (int i = 1; i <= n; i++) {
for (int j = 1; j <= m; j++) {
cin >> maze[i][j];
}
}
dfs(1, 1, -1, -1, 'V');
for (const auto& p : path) {
if (p.second == 1) {
cout << '\n';
}
if (maze[p.first][p.second] == 'V') {
cout << 'L';
} else if (maze[p.first][p.second] == 'H') {
cout << 'H';
} else if (maze[p.first][p.second] == 'W') {
cout << 'W';
}
}
return 0;
}
```
在本程序中,我们使用一个visited数组来记录哪些格子已经走过,并使用一个path数组来记录当前路径。dfs函数的参数x和y表示当前所在的位置,px和py表示上一个位置,dir表示方向。当我们从一个格子走到下一个格子时,如果方向改变了,就将当前格子标记为W。最后,我们遍历path数组,输出每个格子的标记。
阅读全文
相关推荐
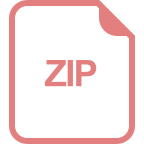
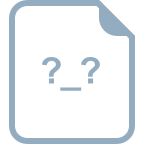

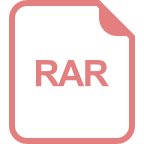
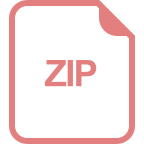
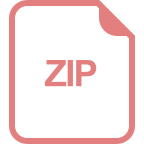
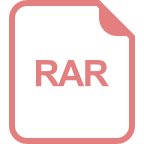
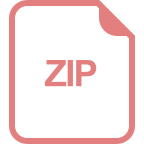
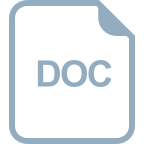
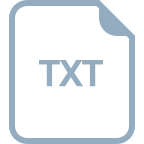
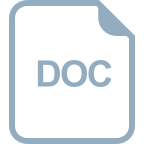


