java图形界面如何实现窗口界面,窗口界面里面有用户名和密码、登陆和取消
时间: 2024-01-17 11:03:50 浏览: 32
要实现一个窗口界面,需要使用Java的Swing或JavaFX等图形界面库。下面是一个使用Swing实现的窗口界面,其中包含用户名和密码输入框、登陆和取消按钮:
```
import javax.swing.*;
import java.awt.*;
import java.awt.event.*;
public class LoginWindow extends JFrame implements ActionListener {
private JTextField usernameField;
private JPasswordField passwordField;
private JButton loginButton;
private JButton cancelButton;
public LoginWindow() {
super("Login");
setDefaultCloseOperation(JFrame.EXIT_ON_CLOSE);
setLayout(new BorderLayout());
// 创建输入框和按钮
JPanel inputPanel = new JPanel(new GridLayout(2, 2));
JLabel usernameLabel = new JLabel("Username: ");
usernameField = new JTextField();
JLabel passwordLabel = new JLabel("Password: ");
passwordField = new JPasswordField();
inputPanel.add(usernameLabel);
inputPanel.add(usernameField);
inputPanel.add(passwordLabel);
inputPanel.add(passwordField);
add(inputPanel, BorderLayout.CENTER);
JPanel buttonPanel = new JPanel(new FlowLayout());
loginButton = new JButton("Login");
loginButton.addActionListener(this);
cancelButton = new JButton("Cancel");
cancelButton.addActionListener(this);
buttonPanel.add(loginButton);
buttonPanel.add(cancelButton);
add(buttonPanel, BorderLayout.SOUTH);
// 显示窗口
pack();
setLocationRelativeTo(null);
setVisible(true);
}
// 处理按钮点击事件
public void actionPerformed(ActionEvent e) {
if (e.getSource() == loginButton) {
String username = usernameField.getText();
String password = new String(passwordField.getPassword());
// 在这里处理登陆逻辑
System.out.println("Username: " + username);
System.out.println("Password: " + password);
} else if (e.getSource() == cancelButton) {
// 在这里处理取消逻辑
System.out.println("Cancel");
}
dispose();
}
public static void main(String[] args) {
new LoginWindow();
}
}
```
在这个例子中,我们创建了一个继承自JFrame的LoginWindow类,它包含了一个用户名输入框、一个密码输入框和两个按钮。在构造函数中,我们创建了这些组件并设置了它们的布局。在按钮被点击时,我们通过实现ActionListener接口处理了按钮事件。在这里,我们简单地输出了用户名和密码,你需要在这里添加你自己的登陆逻辑。最后,我们通过调用setVisible(true)方法显示窗口。
相关推荐
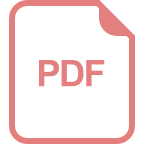
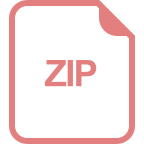
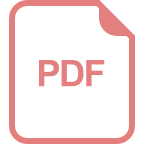














