图片旋转python
时间: 2023-10-29 12:55:56 浏览: 80
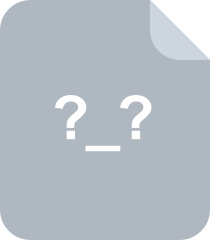
python 实现旋转图像
要在Python中旋转图片,可以使用PIL库(Python Imaging Library)。下面是一个示例代码,演示了如何将横图转为竖图和将竖图转为横图:
```python
from PIL import Image
def rotate_image(image_path, output_path):
with Image.open(image_path) as image:
if image.width > image.height:
# 横图,需要旋转
rotated_image = image.transpose(method=Image.ROTATE_270)
rotated_image.save(output_path)
else:
# 竖图,不需要操作
image.save(output_path)
# 示例用法
image_path = "path/to/image"
output_path = "path/to/output"
rotate_image(image_path, output_path)
```
如果要实现水平翻转和垂直翻转,可以使用以下示例代码:
```python
from PIL import Image
def flip_horizontal(image_path, output_path):
with Image.open(image_path) as image:
flipped_image = image.transpose(method=Image.FLIP_LEFT_RIGHT)
flipped_image.save(output_path)
def flip_vertical(image_path, output_path):
with Image.open(image_path) as image:
flipped_image = image.transpose(method=Image.FLIP_TOP_BOTTOM)
flipped_image.save(output_path)
```
上述代码中,`flip_horizontal`和`flip_vertical`分别是水平翻转和垂直翻转的函数。`image_path`是输入图片的路径,`output_path`是输出图片的路径。你可以根据需要选择其中一种或两种方式来进行图片旋转和翻转操作。
阅读全文
相关推荐
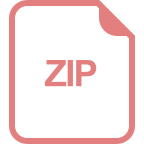














