input1 = Input(shape=(trainX1.shape[1], trainX1.shape[2],)) timesteps1 = trainX1.shape[1] features1 = trainX1.shape[2] # 定义输入层 input_seq1 = Input(shape=(None, timesteps1)) # 时序输入 input_vec1 = Input(shape=(features1,)) # 维度输入 # 定义LSTM层 lstm_output1 = LSTM(units=21, return_sequences=True)(input_seq1) # 返回每个时刻的输出 # 定义attention层 score1 = Dot(axes=[2, 1])([lstm_output1, input_vec1]) # 计算注意力分数 attention_weights1 = Dense(units=1, activation='softmax')(score1) # 计算注意力权重 context_vector1 = Dot(axes=[1, 1])([lstm_output1, attention_weights1]) # 计算上下文向量 # 定义输出层 output1 = concatenate([context_vector1, input_vec1]) output1 = Dense(units=32, activation='relu')(output1) output1 = Dense(units=1, activation='sigmoid')(output1) 出错 如下: File "C:\Users\200511672\Anaconda3\lib\site-packages\keras\initializers.py", line 209, in __call__ scale /= max(1., float(fan_in + fan_out) / 2) TypeError: unsupported operand type(s) for +: 'NoneType' and 'int'
时间: 2023-11-11 22:06:42 浏览: 36
这个错误通常是由于输入形状中的未知维度引起的。在你的代码中,`input_seq1`的第二个维度是`None`,这意味着它可以接受不同长度的序列。但是,在定义LSTM层时,你需要指定时间步长和特征维度。因此,你需要在定义LSTM层时指定时间步长和特征维度。你可以尝试以下修改:
```python
input_seq1 = Input(shape=(timesteps1, features1)) # 时序输入
```
然后,确保你的训练数据`trainX1`的形状是`(样本数, 时间步长, 特征维度)`。如果`trainX1`的时间步长是可变的,则需要对序列进行填充以使其具有相同的长度。你可以使用Keras的`pad_sequences()`函数来实现填充。
相关问题
input_data = Input(shape=(trainX1.shape[1], trainX1.shape[2],)) timesteps = trainX1.shape[1] features = trainX1.shape[2] # 计算时间步的注意力权重 attention_probs1 = Dense(timesteps, activation='softmax')(input_data) attention_probs1 = Permute((2, 1))(attention_probs1) # 将注意力权重应用于输入数据 attention_mul1 = multiply([input_data, attention_probs]) attention_mul1 = Lambda(lambda x: K.sum(x, axis=1))(attention_mul1) # 计算维的注意力权重 attention_probs2 = Dense(INPUT_DIM, activation='softmax')(input_data) attention_probs2 = Permute((2, 1))(attention_probs2) # 将注意力权重应用于输入数据 attention_mul2 = multiply([input_data, attention_probs2]) attention_mul2 = Lambda(lambda x: K.sum(x, axis=1))(attention_mul2) 如何链接attention_mul1和attention_mul2
可以使用K.concatenate()函数将两个注意力向量连接起来,如下所示:
```
merged_vector = K.concatenate([attention_mul1, attention_mul2])
```
这将返回一个连接了两个注意力向量的张量。
1dcnn batch_input_shape
1dcnn的batch_input_shape参数是指定1D卷积神经网络(1D CNN)输入数据的批量大小和形状。在1D CNN中,输入数据通常是一维的序列数据,比如文本或音频信号。
batch_input_shape是一个元组,指定了输入数据的维度,通常为(batch_size, timesteps, input_dim)。其中,batch_size表示每个训练批次的样本数量,timesteps表示序列数据的长度,input_dim表示每个时间步的特征维度。
通过设置batch_size,我们可以同时输入多个样本进行训练。较大的批量大小可以加快训练速度,但也会增加内存消耗。较小的批量大小可能会导致训练不稳定,对噪声更敏感。
timesteps表示1D CNN的输入序列长度。对于文本分类任务,timesteps通常表示文本的单词数量;对于音频分类任务,timesteps表示音频信号的采样点数量。合适的timesteps取决于具体问题,需要根据数据集的特点进行选择。
input_dim表示每个时间步的特征维度。对于文本分类任务,可以使用词嵌入技术将每个单词映射为固定维度的向量表示;对于音频分类任务,可以使用音频信号的特征提取方法(如MFCC)将每个采样点转换为固定维度的特征向量。
通过正确设置1D CNN的batch_input_shape,我们可以将输入数据与模型架构相对应,以便训练和预测。这个参数非常重要,需要仔细考虑,以确保模型能够有效地处理所需的数据和任务。
相关推荐
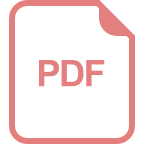
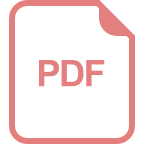












