ValueError: Input 0 of layer lstm_2 is incompatible with the layer: expected ndim=3, found ndim=2. Full shape received: (None, 32)
时间: 2024-03-17 15:43:24 浏览: 19
这个错误通常是由于输入数据的维度与 LSTM 层所期望的不同导致的。LSTM 层的输入应该是三维的,形如 (batch_size, timesteps, input_dim),但是你的输入数据的维度只有两维,形如 (batch_size, input_dim)。
你需要将输入数据转换为三维的形式。你可以使用 `tf.expand_dims` 函数来添加一个新的维度,或者使用 `tf.reshape` 函数将输入数据重塑为三维形式。
例如,对于形如 (batch_size, input_dim) 的输入数据,你可以使用以下代码将其转换为形如 (batch_size, 1, input_dim) 的三维数据:
```python
import tensorflow as tf
input_data = ... # 形如 (batch_size, input_dim) 的输入数据
input_data = tf.expand_dims(input_data, axis=1)
```
或者你可以使用 `tf.reshape` 函数来重塑输入数据的形状:
```python
import tensorflow as tf
input_data = ... # 形如 (batch_size, input_dim) 的输入数据
input_data = tf.reshape(input_data, (batch_size, 1, input_dim))
```
相关问题
ValueError: Input 0 of layer "lstm" is incompatible with the layer: expected ndim=3, found ndim=2. Full shape received: (None, 10)
这个错误是由于LSTM层期望输入的维度是三维的,但实际上输入的维度只有两维导致的。你需要将输入数据从二维转换为三维。可以使用 np.expand_dims() 函数来添加一个新的维度。例如,如果你的输入数据是一个形状为 (batch_size, timesteps) 的二维数组,可以使用以下代码将其转换为形状为 (batch_size, timesteps, input_dim) 的三维数组:
```
import numpy as np
# 输入数据的形状为 (batch_size, timesteps)
input_data = np.random.randn(32, 10)
# 添加一个新的维度,形状为 (batch_size, timesteps, 1)
input_data = np.expand_dims(input_data, axis=-1)
# 现在输入数据的形状为 (batch_size, timesteps, 1),可以传递给 LSTM 层了
```
在实际使用中,你需要将你的数据按照上述方式转换为三维数组,然后再传递给 LSTM 层。
ValueError: Input 0 of layer sequential is incompatible with the layer: expected ndim=3, found ndim=2. Full shape received: (None, 10)怎么修改代码
这个错误通常是由于输入数据维度不匹配导致的。你可以检查一下输入数据的维度是否正确。如果输入数据的维度是 `(batch_size, sequence_length)`,而模型期望的输入维度应该是 `(batch_size, sequence_length, embedding_size)`,则会出现这个错误。
你可以在模型的第一层添加一个 `Input` 层,并指定正确的 `input_shape` 参数。例如,如果你的输入数据维度是 `(batch_size, sequence_length)`,而嵌入层的维度是 `embedding_size`,则可以这样定义输入层:
```
from tensorflow.keras.layers import Input, Embedding, LSTM, Dense
from tensorflow.keras.models import Model
input_layer = Input(shape=(sequence_length,))
embedding_layer = Embedding(input_dim=vocab_size, output_dim=embedding_size)(input_layer)
lstm_layer = LSTM(units=hidden_size)(embedding_layer)
output_layer = Dense(units=output_size, activation='softmax')(lstm_layer)
model = Model(inputs=input_layer, outputs=output_layer)
```
在这个例子中,我们首先定义了一个输入层 `input_layer`,并指定了 `input_shape=(sequence_length,)`。然后我们添加了一个嵌入层 `embedding_layer`,它的输入是 `input_layer` 的输出,输出维度是 `embedding_size`。接下来我们添加了一个 LSTM 层 `lstm_layer`,它的输入是 `embedding_layer` 的输出,输出维度是 `hidden_size`。最后我们添加了一个输出层 `output_layer`,它的输入是 `lstm_layer` 的输出,输出维度是 `output_size`,激活函数是 `softmax`。
这样定义模型之后,你可以使用 `model.fit()` 方法来训练模型。在训练之前,你需要将输入数据转换成正确的维度,例如,如果你有一个形状为 `(batch_size, sequence_length)` 的输入数据张量 `x`,你可以这样将它转换成 `(batch_size, sequence_length, embedding_size)` 的张量:
```
x = np.random.randint(0, vocab_size, size=(batch_size, sequence_length))
x = embedding_layer(x)
```
相关推荐
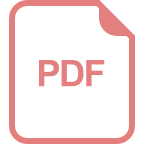
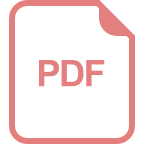
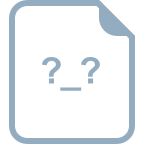









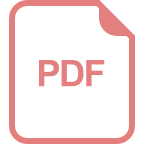
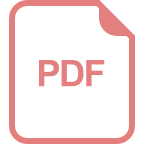
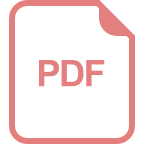
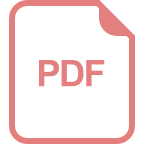