如何用Devc++编写一个飞行棋游戏的代码
时间: 2023-12-06 10:04:37 浏览: 42
以下是使用Dev-C++编写一个简单的飞行棋游戏的代码示例:
```c++
#include <iostream>
#include <cstdlib>
#include <ctime>
using namespace std;
const int BOARDSIZE = 56;
const int PLAYER_NUM = 4;
struct Player {
int position;
int steps;
bool finished;
};
void initBoard(int board[]) {
for (int i = 0; i < BOARDSIZE; i++) {
board[i] = 0;
}
board[0] = board[13] = board[26] = board[39] = 1; // 红色区域
board[9] = board[22] = board[35] = board[48] = 2; // 蓝色区域
board[18] = board[31] = board[44] = board[3] = 3; // 黄色区域
board[27] = board[40] = board[51] = board[14] = 4; // 绿色区域
}
void rollDice(int &dice) {
dice = rand() % 6 + 1;
}
void initPlayers(Player players[]) {
for (int i = 0; i < PLAYER_NUM; i++) {
players[i].position = -1;
players[i].steps = 0;
players[i].finished = false;
}
}
void move(Player &player, int steps, int board[]) {
int next_pos = player.position + steps;
if (next_pos >= BOARDSIZE) { // 超出终点
player.position = BOARDSIZE - 1;
player.finished = true;
return;
}
if (board[next_pos] != 0) { // 遇到其他棋子
player.position = next_pos;
return;
}
board[player.position] = 0; // 清除原位置
player.position = next_pos;
board[player.position] = 1; // 标记新位置
}
void printBoard(int board[]) {
for (int i = 0; i < BOARDSIZE; i++) {
cout << board[i] << " ";
if ((i + 1) % 14 == 0) {
cout << endl;
}
}
}
int main() {
srand(time(0));
int board[BOARDSIZE];
initBoard(board);
Player players[PLAYER_NUM];
initPlayers(players);
int cur_player = 0;
int dice;
while (true) {
cout << "Player " << cur_player + 1 << "'s turn. Press any key to roll the dice." << endl;
cin.get();
rollDice(dice);
cout << "You rolled " << dice << "." << endl;
if (players[cur_player].finished) {
cout << "You have finished the game." << endl;
} else {
move(players[cur_player], dice, board);
cout << "You move to position " << players[cur_player].position << "." << endl;
if (players[cur_player].finished) {
cout << "You have finished the game." << endl;
}
}
printBoard(board);
cur_player = (cur_player + 1) % PLAYER_NUM;
}
return 0;
}
```
这个代码实现了一个简单的飞行棋游戏,由四个玩家逐个轮流掷骰子并移动棋子。其中,initBoard函数初始化了游戏棋盘,initPlayers函数初始化了玩家信息,rollDice函数掷骰子,move函数移动棋子,printBoard函数打印当前棋盘状态。
相关推荐
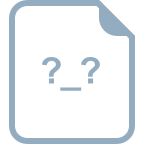
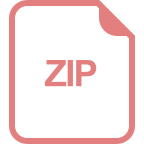














