创建顺序栈,求栈中的数据元素个数,将已经存在的栈置成空在顺序栈上实现入栈,出栈等操作,JAVA
时间: 2024-10-12 21:08:31 浏览: 39
在Java中,我们可以使用数组(Array)或动态数组(ArrayList)来创建简单的顺序栈。这里我会展示如何使用数组实现,因为这是基础概念。如果你有特定的问题或者使用了某种特定的数据结构,比如LinkedList,也请告诉我。
**顺序栈实现:**
```java
public class SimpleStack {
private int[] stack; // 数组用于存储栈元素
private int top; // 栈顶指针
// 构造器,初始化栈容量
public SimpleStack(int capacity) {
stack = new int[capacity];
top = -1;
}
// 检查栈是否为空
public boolean isEmpty() {
return top == -1;
}
// 判断栈是否已满
public boolean isFull() {
return top == stack.length - 1;
}
// 入栈操作 (push)
public void push(int value) {
if (!isFull()) {
top++;
stack[top] = value;
} else {
System.out.println("Stack is full, cannot push.");
}
}
// 出栈操作 (pop)
public int pop() {
if (!isEmpty()) {
int poppedValue = stack[top];
top--;
return poppedValue;
} else {
System.out.println("Stack is empty, cannot pop.");
return -1; // 返回默认值,防止程序错误
}
}
// 获取栈顶元素但不删除 (peek)
public int peek() {
if (!isEmpty()) {
return stack[top];
} else {
System.out.println("Stack is empty.");
return -1; // 返回默认值
}
}
// 将栈清空 (clear)
public void clear() {
top = -1;
}
}
```
**使用示例:**
```java
SimpleStack myStack = new SimpleStack(5);
myStack.push(1); // 入栈
System.out.println(myStack.peek()); // 输出:1
myStack.push(2);
System.out.println(myStack.pop()); // 输出:1
System.out.println(myStack.isEmpty()); // 输出:false
myStack.clear(); // 清空栈
```
阅读全文
相关推荐
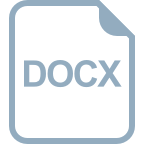
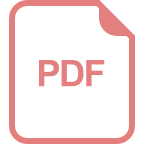
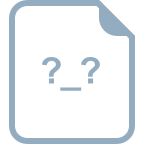
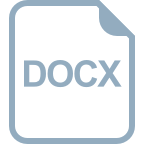
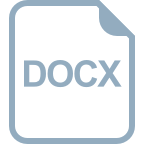
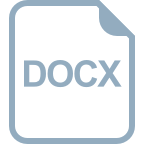
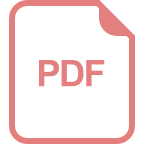
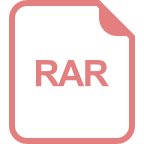
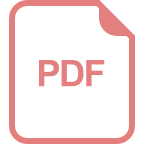
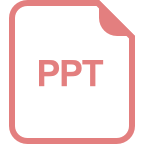
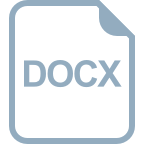
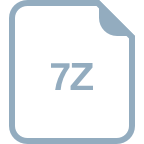
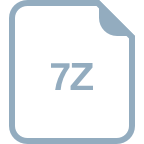
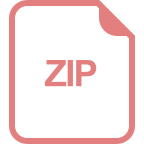
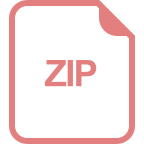