java实现LR(0)活前缀DFA的构造,输入一个普通的文法 ,输出输入文法的活前缀DFA
时间: 2024-02-27 22:52:11 浏览: 116
好的,我明白了。LR(0) 活前缀 DFA 的构造可以分为以下几个步骤:
1. 对输入的文法进行扩充,添加一个起始符号 S',将 S' -> S 作为第一条产生式。
2. 构造 LR(0) 项集族,即每个项集表示了从某个状态经过某个符号可以转移到的所有状态。
3. 对每个 LR(0) 项集进行闭包运算,即将所有可能的后继符号加入该项集中,直到没有新的符号可以加入为止。
4. 对每个 LR(0) 项集进行转移运算,即对每个非终结符构造一个新的项集,表示从当前项集经过该非终结符可以转移到的所有状态。
5. 对所有项集进行编号,作为状态标识。
6. 构造活前缀 DFA,即对每个状态和每个终结符构造一个转移边,表示从当前状态经过该终结符可以转移到的下一个状态。
下面给出 Java 代码实现:
```java
import java.util.*;
public class LR0DFA {
private final Grammar grammar; // 输入文法
private final Map<LR0State, Integer> stateMap; // 状态到编号的映射
private final List<LR0State> stateList; // 状态列表
private final List<Map<String, Integer>> transitionTable; // 转移表
public LR0DFA(Grammar grammar) {
this.grammar = grammar;
this.stateMap = new HashMap<>();
this.stateList = new ArrayList<>();
this.transitionTable = new ArrayList<>();
build();
}
// 构造 LR(0) 活前缀 DFA
private void build() {
// 对文法进行扩充
String startSymbol = grammar.getStartSymbol();
Production augmentedProduction = new Production("S'", Collections.singletonList(startSymbol));
List<Production> productions = new ArrayList<>(grammar.getProductions());
productions.add(0, augmentedProduction);
Grammar augmentedGrammar = new Grammar(grammar.getTerminals(), grammar.getNonTerminals(), startSymbol, productions);
// 构造 LR(0) 项集族
LR0State startState = new LR0State(augmentedGrammar, augmentedProduction, 0);
Set<LR0State> stateSet = new HashSet<>();
stateSet.add(startState);
Queue<LR0State> queue = new LinkedList<>();
queue.offer(startState);
while (!queue.isEmpty()) {
LR0State state = queue.poll();
for (String symbol : state.getNextSymbols()) {
LR0State nextState = state.go(symbol);
if (!stateSet.contains(nextState)) {
stateSet.add(nextState);
queue.offer(nextState);
}
}
}
// 对每个 LR(0) 项集进行闭包和转移运算
for (LR0State state : stateSet) {
state.closure();
}
for (LR0State state : stateSet) {
Map<String, LR0State> nextMap = state.getNextStateMap();
Map<String, Integer> transitionMap = new HashMap<>();
for (Map.Entry<String, LR0State> entry : nextMap.entrySet()) {
String symbol = entry.getKey();
LR0State nextState = entry.getValue();
if (!stateSet.contains(nextState)) {
continue;
}
int nextStateId = stateMap.computeIfAbsent(nextState, s -> stateList.size());
transitionMap.put(symbol, nextStateId);
}
stateList.add(state);
stateMap.put(state, stateList.size() - 1);
transitionTable.add(transitionMap);
}
}
// 获取状态数
public int getStateCount() {
return stateList.size();
}
// 获取转移表
public List<Map<String, Integer>> getTransitionTable() {
return transitionTable;
}
// 获取状态对应的编号
public int getStateId(LR0State state) {
return stateMap.get(state);
}
}
```
其中,LR0State 表示 LR(0) 项集,包含了该项集中的所有项以及从该项集可以转移到的下一个状态。LR0State 的 go 方法表示对该项集进行转移运算,返回一个新的项集。LR0State 的 closure 方法表示对该项集进行闭包运算,将所有可能的后继符号加入该项集中。
转移表是一个二维列表,列表的每个元素是一个 Map,表示某个状态在某个终结符下可以转移到的下一个状态的编号。状态通过 stateMap 进行编号,编号从 0 开始递增。getStateCount 方法返回状态数,getStateId 方法返回某个状态对应的编号。
使用 LR0DFA 类可以对输入的文法构造 LR(0) 活前缀 DFA,获取状态数和转移表。例如,对于以下文法:
```
S -> E
E -> E + T | T
T -> int * T | int
```
可以这样使用 LR0DFA 类:
```java
Grammar grammar = new Grammar(
Arrays.asList("int", "+", "*"),
Arrays.asList("S", "E", "T"),
"S",
Arrays.asList(
new Production("S", Collections.singletonList("E")),
new Production("E", Arrays.asList("E", "+", "T")),
new Production("E", Collections.singletonList("T")),
new Production("T", Arrays.asList("int", "*", "T")),
new Production("T", Collections.singletonList("int"))
)
);
LR0DFA dfa = new LR0DFA(grammar);
int stateCount = dfa.getStateCount();
List<Map<String, Integer>> transitionTable = dfa.getTransitionTable();
```
这样,stateCount 就是状态数,transitionTable 就是转移表。
阅读全文
相关推荐
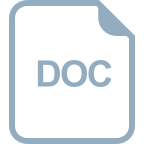










