为防止接口被恶意调用,设计 API 秘钥、令牌校验等方式来提高接口安全性,并通过滑动窗口实现接口调用限流 怎么用java实现
时间: 2024-11-18 10:19:28 浏览: 29
为了提高API接口的安全性并实施滑动窗口限流,可以采用以下步骤在Java中实现:
1. **设计API秘钥和令牌校验**[^1]:
- 使用OAuth 2.0或者JWT(JSON Web Tokens)进行身份验证。创建一个API密钥,客户端请求时携带该密钥进行认证。
```java
// 示例使用Spring Security的TokenProvider
@Bean
public JwtAuthenticationTokenFilter jwtAuthenticationTokenFilter() {
return new JwtAuthenticationTokenFilter(jwtConfig());
}
```
2. **滑动窗口计数器**[^2]:
- 实现一个基于时间窗口的限流策略,如Redis中的有序集合(Sorted Set)存储每个API key的最后访问时间和调用次数。
```java
import org.springframework.data.redis.core.RedisTemplate;
import org.springframework.stereotype.Component;
@Component
public class RateLimiter {
private final String apiKeySetKey = "api_key_window";
private final RedisTemplate<String, Object> redisTemplate;
public boolean allowAccess(String apiKey, long currentTime) {
// 获取当前时间戳内的调用次数
Long count = redisTemplate.opsForValue().get(apiKeySetKey + "_" + currentTime);
if (count == null) {
count = 0;
}
// 检查是否超过阈值
if (count >= maxRequestsPerWindow) {
return false;
} else {
// 更新计数并过期时间
redisTemplate.opsForValue().set(apiKeySetKey + "_" + currentTime, count + 1, windowDuration, TimeUnit.SECONDS);
return true;
}
}
}
```
阅读全文
相关推荐
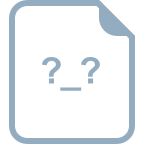
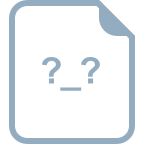
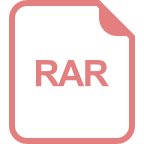

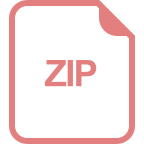
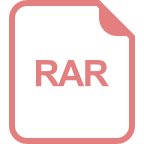
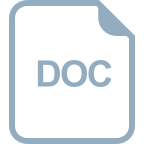
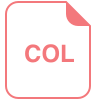
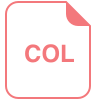
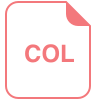
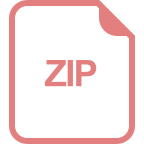
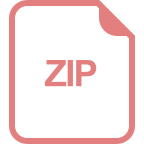
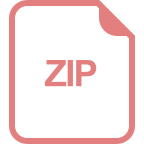
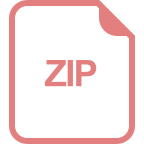
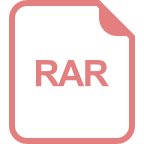
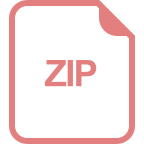
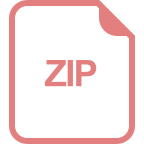
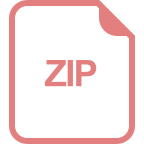
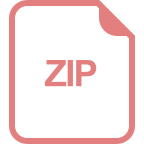