function sysCall_init() forwarder=sim.getObjectHandle('ConveyorBelt_forwarder') sim.setFloatSignal("belt_con",1) sen=sim.getObjectHandle('sensor') end function sysCall_cleanup() end function sysCall_actuation() beltVelocity=sim.getScriptSimulationParameter(sim.handle_self,"conveyorBeltVelocity") con=sim.getFloatSignal("belt_con") senflag,dis,_,_,_=sim.readProximitySensor(sen) print(senflag) if con==1 and senflag ==0 then -- Here we "fake" the transportation pads with a single static rectangle that we dynamically reset -- at each simulation pass (while not forgetting to set its initial velocity vector) : relativeLinearVelocity={beltVelocity,0,0} -- Reset the dynamic rectangle from the simulation (it will be removed and added again) sim.resetDynamicObject(forwarder) -- Compute the absolute velocity vector: m=sim.getObjectMatrix(forwarder,-1) m[4]=0 -- Make sure the translation component is discarded m[8]=0 -- Make sure the translation component is discarded m[12]=0 -- Make sure the translation component is discarded absoluteLinearVelocity=sim.multiplyVector(m,relativeLinearVelocity) -- Now set the initial velocity of the dynamic rectangle: sim.setObjectFloatParameter(forwarder,sim.shapefloatparam_init_velocity_x,absoluteLinearVelocity[1]) sim.setObjectFloatParameter(forwarder,sim.shapefloatparam_init_velocity_y,absoluteLinearVelocity[2]) sim.setObjectFloatParameter(forwarder,sim.shapefloatparam_init_velocity_z,absoluteLinearVelocity[3]) end end
时间: 2024-04-01 15:38:32 浏览: 196
这段代码是用来控制一个传送带的运行的,其中使用了一个名为 forwarder 的物体来模拟传送带的运动,同时使用了一个名为 sen 的物体来检测传送带上是否存在物体。在代码中通过 sim.getFloatSignal("belt_con") 获取到传送带的控制信号,如果该信号为 1 并且传送带上没有物体,则通过 sim.resetDynamicObject(forwarder) 重置 forwarder 物体,并使用 sim.setObjectFloatParameter() 设置其初速度,从而实现传送带的运动。
相关问题
function sysCall_init() -- do some initialization here dirh={-1,-1,-1,-1} speedh={-1,-1,-1,-1} dirh[1]=sim.getObjectHandle('carwdfl') dirh[2]=sim.getObjectHandle('carwdfr') dirh[3]=sim.getObjectHandle('carwdbl') dirh[4]=sim.getObjectHandle('carwdbr') speedh[1]=sim.getObjectHandle('carwfl') speedh[2]=sim.getObjectHandle('carwfr') speedh[3]=sim.getObjectHandle('carwbl') speedh[4]=sim.getObjectHandle('carwbr') car_aim_h=sim.getObjectHandle('car_aim') car_now_h=sim.getObjectHandle('car_now') car_f_h=sim.getObjectHandle('car_f') car_w_speed={0,0,0,0} direrr=-math.pi/2 end function sysCall_actuation() -- put your actuation code here car_aim=sim.getObjectPosition(car_aim_h,-1) car_f=sim.getObjectPosition(car_now_h,-1) car_now=sim.getObjectPosition(car_f_h,-1) yerr=car_aim[2]-car_now[2] xerr=car_aim[1]-car_now[1] ycarf=car_now[2]-car_f[2] xcarf=car_now[1]-car_f[1] car_w_speed={0,0,1.5,1} aimangle=math.atan2(yerr,xerr) carangle=math.atan2(ycarf,xcarf) rerr=carangle-aimangle xyerr=yerr^2+xerr^2 print(xyerr) if xyerr>0.01 then vf=-1 else vf=0 end --[[ if math.abs(rerr)>0.01 then vr=1*rerr/math.abs(rerr) vf=0 else vr=0 end if 1 then car_w_speed[3]=vr+vf car_w_speed[4]=-vr+vf end --]] for ii=1,4,1 do sim.setJointTargetPosition(dirh[ii],-rerr+direrr) sim.setJointTargetVelocity(speedh[ii],vf) end end function sysCall_sensing() -- put your sensing code here end function sysCall_cleanup() -- do some clean-up here end -- See the user manual or the available code snippets for additional callback functions and details
这是一个用于Simulink仿真环境的Lua脚本,主要用于控制一个四轮驱动的小车朝向目标点行驶。在每个时间步长内,脚本将从仿真环境中获取小车当前位置和目标点位置,计算当前位置与目标点之间的水平距离和垂直距离,然后将小车的方向调整到指向目标点。具体来说,脚本会将小车的前后左右四个轮子的角度和速度进行调整,使得小车能够向目标点前进。需要注意的是,这个脚本中的代码并没有完全实现,部分代码被注释掉了。如果你想要使用这个脚本,需要根据自己的需要进行修改和完善。
#define __SYSCALL_NARGS_X(a,b,c,d,e,f,g,h,n,...) n #define __SYSCALL_NARGS(...) __SYSCALL_NARGS_X(__VA_ARGS__,7,6,5,4,3,2,1,0,)
这个宏定义`__SYSCALL_NARGS_X`和`__SYSCALL_NARGS`是用来计算可变参数列表中参数的数量的。它通常在编写操作系统或系统调用函数中使用,特别是那些需要动态处理不同数量参数的情况。
`__SYSCALL_NARGS_X`是一个模板函数,通过`__VA_ARGS__`语法接受任意数量的参数,并通过一系列占位符(如7,6,5等)检查参数是否存在,直到找到实际的结束标记`0,`。当所有参数都被检查完,宏会返回最后一个占位符对应的数值,也就是实际参数的个数。
`__SYSCALL_NARGS`则是一个宏展开后的表达式,用于在实际代码中调用`__SYSCALL_NARGS_X`并获取最终结果。当我们在代码中看到`__SYSCALL_NARGS(...)`的形式,它会被替换为`__SYSCALL_NARGS_X`的实际调用。
例如,如果我们有如下调用:
```cpp
#define MY_SYSCALL(a, b, c, d) __SYSCALL_NARGS(a, b, c, d)
MY_SYSCALL(1, 2, 3)
```
宏展开后,`MY_SYSCALL`会被替换成`__SYSCALL_NARGS_X(1, 2, 3, 0)`,进而计算出实参总数为3。
阅读全文
相关推荐
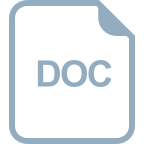
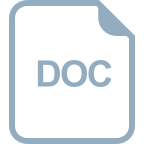
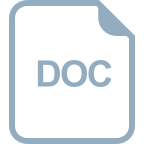


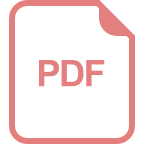
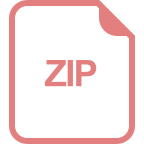
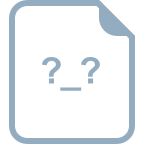
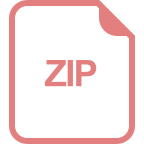
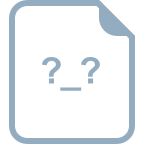
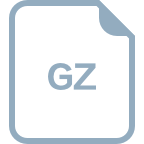





