package main /* #include <sys/socket.h> #include <netpacket/packet.h> #include <net/ethernet.h> */ import "C" import ( "fmt" "log" "net" "os" "os/exec" "syscall" "unsafe" ) const ( ProtocolIP = 0x0800 ETH_P_ALL = 0x0003 BufferSize = 65536 ) var Interface string // 网卡接口名称 func main() { // 检查程序是否以root身份运行 if os.Geteuid() != 0 { fmt.Println("Please run the program as root.") // 以root身份重新运行程序 cmd := exec.Command("sudo", os.Args[0]) cmd.Stdout = os.Stdout cmd.Stdin = os.Stdin cmd.Stderr = os.Stderr err := cmd.Run() if err != nil { log.Fatal(err) } os.Exit(0) } // 获取系统的网络接口信息 interfaces, err := net.Interfaces() if err != nil { log.Fatal(err) } // 遍历网络接口,打印接口名称 fmt.Println("Available network interfaces:") for _, iface := range interfaces { fmt.Println(iface.Name) } // 设置要使用的网卡接口(例如:eth0) Interface = "eth0" // 更改为你的网卡接口名 // 创建原始套接字 sockFD, err := syscall.Socket(C.AF_PACKET, syscall.SOCK_RAW, int(htons(ETH_P_ALL))) if err != nil { log.Fatal(err) } defer syscall.Close(sockFD) // 获取网卡接口索引 iface, err := net.InterfaceByName(Interface) if err != nil { log.Fatal(err) } // 绑定原始套接字到网卡接口 sa := C.struct_sockaddr_ll{ sll_family: C.AF_PACKET, sll_protocol: htons(ETH_P_ALL), sll_ifindex: C.int(iface.Index), } if err := syscall.Bind(sockFD, (*syscall.Sockaddr)(unsafe.Pointer(&sa))); err != nil { log.Fatal(err) } // 在一个无限循环中接收数据包 for { buffer := make([]byte, BufferSize) n, _, err := syscall.Recvfrom(sockFD, buffer, 0) if err != nil { log.Fatal(err) } fmt.Printf("Received packet: %s\n", string(buffer[:n])) } } func htons(i uint16) uint16 { return (i<<8)&0xff00 | i>>8 }中无法将 '(*syscall.Sockaddr)(unsafe.Pointer(&sa))' (类型 *syscall.Sockaddr) 用作类型 Sockaddr
时间: 2024-04-11 12:32:08 浏览: 183
这段代码是一个使用原始套接字进行数据包捕获的示例。在这段代码中,使用了C语言的头文件和函数来实现底层的网络操作。
在Go语言中,`(*syscall.Sockaddr)(unsafe.Pointer(&sa))` 这一部分是将 `sa` 转换为 `syscall.Sockaddr` 类型的指针。但是根据您提供的代码,似乎没有定义 `Sockaddr` 或 `struct_sockaddr_ll` 这些结构体。
如果您想在Go语言中使用原始套接字,可以尝试使用`golang.org/x/net/bpf`包或`github.com/google/gopacket`包,它们提供了更高级的API来处理网络数据包。
请问您有其他问题需要帮助吗?
相关问题
#include <pcap.h> #include <netinet/ip.h> #include <netinet/tcp.h>
在你提供的引用内容中,有关于使用`#include <arpa/inet.h>`头文件的说明。根据这个头文件的说明,我们可以使用其中的函数和结构体,而不需要重新定义。所以,在你提供的代码中,不需要重新定义`struct in_addr`。
以下是使用`#include <pcap.h>`、`#include <netinet/ip.h>`和`#include <netinet/tcp.h>`的示例代码:
```c
#include <pcap.h>
#include <netinet/ip.h>
#include <netinet/tcp.h>
int main() {
// 打开网络设备
pcap_t *handle;
char errbuf[PCAP_ERRBUF_SIZE];
handle = pcap_open_live("eth0", BUFSIZ, 1, 1000, errbuf);
if (handle == NULL) {
printf("Couldn't open device: %s\n", errbuf);
return 1;
}
// 抓取数据包
struct pcap_pkthdr header;
const u_char *packet;
packet = pcap_next(handle, &header);
if (packet == NULL) {
printf("No packet found.\n");
return 1;
}
// 解析IP头部
struct ip *ip_header;
ip_header = (struct ip *)(packet + 14); // 偏移14字节,跳过以太网头部
printf("Source IP: %s\n", inet_ntoa(ip_header->ip_src));
printf("Destination IP: %s\n", inet_ntoa(ip_header->ip_dst));
// 解析TCP头部
struct tcphdr *tcp_header;
tcp_header = (struct tcphdr *)(packet + 14 + ip_header->ip_hl * 4); // 偏移14字节 + IP头部长度
printf("Source Port: %d\n", ntohs(tcp_header->th_sport));
printf("Destination Port: %d\n", ntohs(tcp_header->th_dport));
// 关闭网络设备
pcap_close(handle);
return 0;
}
```
这段代码使用了libpcap库来捕获网络数据包,并解析其中的IP头部和TCP头部。其中,`inet_ntoa()`函数用于将IP地址转换为字符串格式。
#include <Winsock2.h> #include<iostream> #include "pcap.h" #include "stdio.h" #include<time.h> #include <string> #include <fstream> //文件的输入输出; #pragma comment(lib,"ws2_32.lib") #pragma comment(lib,"wpcap.lib") #pragma comment(lib,"Packet.lib") using namespace std;
这段代码看起来是一个网络数据包捕捉的程序,使用了Winsock2和pcap库。其中Winsock2是Windows下的套接字编程API,pcap是网络数据包捕捉库。其他库如stdio.h、time.h、string、fstream等则是用于文件操作的。
代码中的#pragma comment(lib,"ws2_32.lib")、#pragma comment(lib,"wpcap.lib")、#pragma comment(lib,"Packet.lib")是用于告诉编译器需要链接这些库文件。
可以看出,这段代码是用C++编写的。
阅读全文
相关推荐
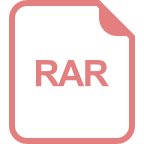
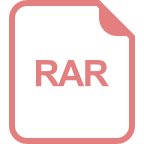
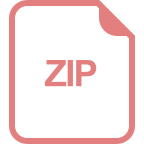
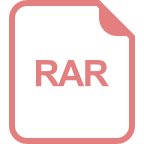
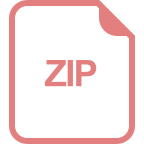
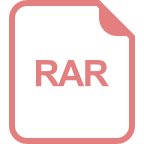
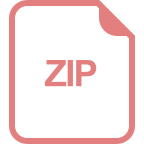
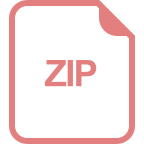
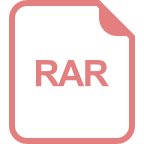
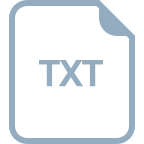
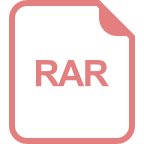
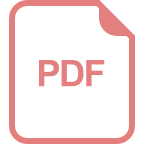