用python实现深度学习模型知识蒸馏
时间: 2024-12-26 22:21:58 浏览: 4
### 使用 Python 实现深度学习模型中的知识蒸馏
#### 背景介绍
知识蒸馏是一种用于提高小型学生模型性能的技术,通过让其模仿大型教师模型的行为。这种方法不仅能够减少计算资源消耗还能保持较高的准确性。
#### 构建教师与学生模型
为了实现这一过程,首先定义两个不同复杂度级别的卷积神经网络作为教师和学生的架构:
```python
import tensorflow as tf
from tensorflow.keras import layers, models
def create_teacher_model():
teacher = models.Sequential([
layers.Conv2D(32, (3, 3), activation='relu', input_shape=(32, 32, 3)),
layers.MaxPooling2D((2, 2)),
layers.Conv2D(64, (3, 3), activation='relu'),
layers.MaxPooling2D((2, 2)),
layers.Flatten(),
layers.Dense(64, activation='relu'),
layers.Dense(10)
])
return teacher
def create_student_model():
student = models.Sequential([
layers.Conv2D(16, (3, 3), activation='relu', input_shape=(32, 32, 3)),
layers.MaxPooling2D((2, 2)),
layers.Flatten(),
layers.Dense(10)
])
return student
```
#### 定义软标签损失函数
接下来创建自定义损失函数以考虑来自教师的概率分布(即所谓的“软目标”),这有助于传递更多关于类间关系的信息给学生模型[^1]:
```python
class Distiller(tf.keras.Model):
def __init__(self, student, teacher):
super(Distiller, self).__init__()
self.teacher = teacher
self.student = student
def compile(self, optimizer, metrics, distillation_loss_fn,
temperature=3):
super(Distiller, self).compile(optimizer=optimizer, metrics=metrics)
self.distillation_loss_fn = distillation_loss_fn
self.temperature = temperature
def train_step(self, data):
# Unpack data
x, y = data
# Forward pass of teacher
teacher_predictions = self.teacher(x, training=False)
with tf.GradientTape() as tape:
# Forward pass of student
student_predictions = self.student(x, training=True)
# Compute loss between soft targets and predictions
distillation_loss = (
self.distillation_loss_fn(
tf.nn.softmax(teacher_predictions / self.temperature),
tf.nn.softmax(student_predictions / self.temperature))
* (self.temperature ** 2))
# Add hard target loss
total_loss = distillation_loss + \
tf.keras.losses.sparse_categorical_crossentropy(y, student_predictions)
# Apply gradients
trainable_vars = self.student.trainable_variables
gradients = tape.gradient(total_loss, trainable_vars)
self.optimizer.apply_gradients(zip(gradients, trainable_vars))
# Update metrics (includes the metric that tracks the loss)
for m in self.metrics:
if m.name == 'loss':
m.update_state(total_loss)
elif m.name == "accuracy":
m.update_state(y, student_predictions)
# Return a dict mapping metric names to current value.
return {m.name: m.result() for m in self.metrics}
```
上述代码展示了如何构建一个简单的`Distiller`类来执行训练逻辑,在这里引入了一个温度参数控制着从硬标签到软标签转换的程度;较低的值更接近于标准交叉熵损失,而较高则倾向于鼓励相似概率分布的学习。
#### 训练并评估模型
完成以上设置之后就可以准备数据集并对模型进行编译、拟合以及最终测试了:
```python
# Prepare dataset...
(train_images, train_labels), (test_images, test_labels) = ...
# Create instances of both architectures
teacher = create_teacher_model()
student = create_student_model()
distiller = Distiller(student=student, teacher=teacher)
distiller.compile(
optimizer=tf.keras.optimizers.Adam(),
metrics=[tf.keras.metrics.SparseCategoricalAccuracy()],
distillation_loss_fn=tf.keras.losses.KLDivergence())
# Train the model using standard keras API calls
history = distiller.fit(...)
# Evaluate performance on unseen samples after completion
results = distiller.evaluate(test_images, test_labels)
print(f'Test accuracy: {results}')
```
这段程序片段说明了整个流程——从初始化对象到最后一步验证结果的质量。值得注意的是实际应用中可能还需要调整超参比如批次大小(batch size),迭代次数(epoch number)等细节因素影响整体效果。
阅读全文
相关推荐
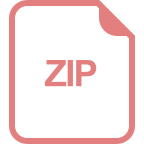
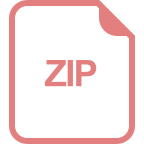
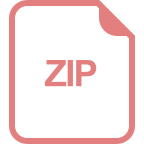
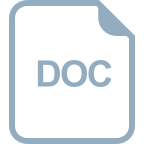
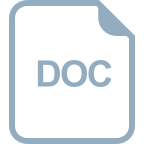
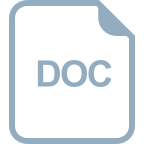
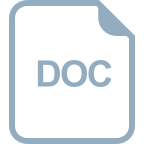
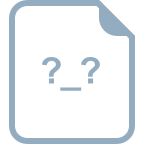
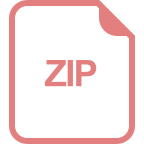
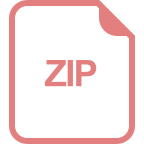
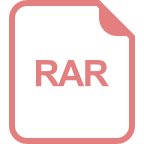
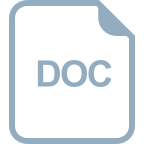
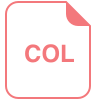
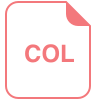
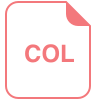
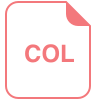
