用MFC写出线性插值函数
时间: 2023-12-17 12:04:00 浏览: 90
下面是使用 MFC 编写的线性插值函数示例代码:
```cpp
// 线性插值函数
double lerp(double x0, double x1, double y0, double y1, double x)
{
return y0 + (y1 - y0) * (x - x0) / (x1 - x0);
}
```
该函数的参数分别为:已知点 x0 和 x1,以及对应的函数值 y0 和 y1,以及插值点 x。函数返回插值点处的函数值。
相关问题
MFC 实现使用线性插值代码
以下是使用MFC实现线性插值的代码示例:
```c++
// 定义线性插值函数
double lerp(double a, double b, double t)
{
return a + (b - a) * t;
}
// 实现线性插值
void CMyAppDlg::OnBnClickedButton1()
{
double x1 = 0.0, y1 = 0.0, x2 = 10.0, y2 = 20.0, x = 5.0, y = 0.0; // 初始化数据
double t = (x - x1) / (x2 - x1); // 计算插值比例
y = lerp(y1, y2, t); // 进行线性插值
CString str;
str.Format(_T("y = %.2f"), y);
SetDlgItemText(IDC_STATIC_RESULT, str);
}
```
在这个示例中,我们定义了一个`lerp()`函数,该函数接受三个参数`a`、`b`和`t`,表示插值的起始点、结束点和插值比例,返回插值结果。然后我们在`OnBnClickedButton1()`函数中初始化了需要进行插值的数据,计算出插值比例`t`,并调用`lerp()`函数进行线性插值,最后将插值结果显示在界面上。
用mfc写出渐变填充函数
以下是一个简单的 MFC 渐变填充函数的示例:
```cpp
void CGradientFill::GradientFill(CDC* pDC, CRect rect, COLORREF colorStart, COLORREF colorEnd, BOOL bHorizontal)
{
// 计算渐变范围
int nWidth = rect.Width();
int nHeight = rect.Height();
// 创建渐变画刷
CBrush brush;
brush.CreateSolidBrush(colorStart);
// 画刷逐渐淡化到 colorEnd
COLORREF color = colorStart;
int rStep = ((colorEnd & 0xFF0000) - (colorStart & 0xFF0000)) / nWidth;
int gStep = ((colorEnd & 0x00FF00) - (colorStart & 0x00FF00)) / nWidth;
int bStep = ((colorEnd & 0x0000FF) - (colorStart & 0x0000FF)) / nWidth;
// 水平渐变
if (bHorizontal)
{
for (int i = 0; i < nWidth; i++)
{
color = RGB(GetRValue(color) + rStep, GetGValue(color) + gStep, GetBValue(color) + bStep);
brush.CreateSolidBrush(color);
pDC->FillRect(CRect(rect.left + i, rect.top, rect.left + i + 1, rect.bottom), &brush);
}
}
// 垂直渐变
else
{
for (int i = 0; i < nHeight; i++)
{
color = RGB(GetRValue(color) + rStep, GetGValue(color) + gStep, GetBValue(color) + bStep);
brush.CreateSolidBrush(color);
pDC->FillRect(CRect(rect.left, rect.top + i, rect.right, rect.top + i + 1), &brush);
}
}
}
```
这个函数接受一个 CDC 对象、矩形区域、起始颜色、结束颜色和一个布尔值,用于指定渐变是水平还是垂直的。函数通过计算每个像素的颜色值来渐变填充矩形区域。
阅读全文
相关推荐
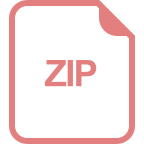
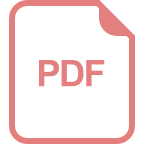
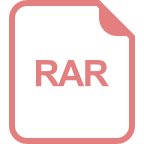













