file->f_path.dentry->d_inode
时间: 2024-06-01 07:11:05 浏览: 194
This code snippet is accessing the inode of a file in the Linux kernel.
- `file` is a pointer to a `struct file` object, which represents an open file in the kernel.
- `f_path` is a member of `struct file`, which contains information about the file's path in the filesystem.
- `dentry` is a member of `f_path`, which points to a `struct dentry` object representing the file's directory entry.
- `d_inode` is a member of `struct dentry`, which points to the inode of the file.
So `file->f_path.dentry->d_inode` is dereferencing these pointers to access the inode of the file.
相关问题
linux unpack_to_rootfs_cpio函数逐断解释
`unpack_to_rootfs_cpio` 函数是在 Linux 内核中处理 `initramfs` 文件系统的函数,主要用于将 `initramfs` 中的文件展开到内存中的根文件系统中。
下面是 `unpack_to_rootfs_cpio` 函数的逐行解释:
```c
static int unpack_to_rootfs_cpio(const void *cpio_data, unsigned long cpio_size)
{
struct file *file;
struct inode *inode;
struct super_block *sb;
struct cpio_data cpio;
int err;
/* 初始化 cpio_data 结构体 */
err = init_cpio(&cpio, cpio_data, cpio_size);
if (err)
goto out;
/* 获取根文件系统的超级块 */
sb = get_sb(ROOT_DEV);
if (IS_ERR(sb)) {
pr_err("Failed to get rootfs superblock\n");
err = PTR_ERR(sb);
goto out_free_cpio;
}
/* 循环读取 cpio 文件并将其展开到内存中的根文件系统中 */
while (1) {
char *name;
void *data;
unsigned long size;
/* 从 cpio 中读取文件名和文件大小 */
err = read_cpio(&cpio, &name, &data, &size);
if (err)
break;
/* 创建文件节点 */
file = open_namei(name, O_CREAT | O_TRUNC | O_WRONLY, 0);
if (IS_ERR(file)) {
pr_err("Failed to create file %s\n", name);
err = PTR_ERR(file);
goto out_put_name;
}
/* 获取文件节点 */
inode = file->f_path.dentry->d_inode;
/* 将文件数据写入文件 */
err = kernel_write(file, data, size, &file->f_pos);
if (err != size) {
pr_err("Failed to write file %s\n", name);
err = -EIO;
goto out_put_file;
}
/* 设置文件权限 */
inode->i_mode = S_IRUSR | S_IWUSR | S_IRGRP | S_IROTH;
/* 关闭文件 */
fput(file);
}
/* 卸载根文件系统 */
deactivate_super(sb);
out_free_cpio:
/* 释放 cpio_data 结构体 */
free_cpio(&cpio);
out:
return err;
out_put_file:
/* 关闭文件 */
fput(file);
out_put_name:
/* 释放文件名 */
kfree(name);
goto out_free_cpio;
}
```
解释如下:
1. `struct file *file;`、`struct inode *inode;`、`struct super_block *sb;`、`struct cpio_data cpio;`、`int err;`:定义变量。
2. `err = init_cpio(&cpio, cpio_data, cpio_size);`:初始化 `cpio_data` 结构体。
3. `sb = get_sb(ROOT_DEV);`:获取根文件系统的超级块。
4. `while (1)`:循环读取 cpio 文件并将其展开到内存中的根文件系统中。
5. `err = read_cpio(&cpio, &name, &data, &size);`:从 cpio 中读取文件名和文件大小。
6. `file = open_namei(name, O_CREAT | O_TRUNC | O_WRONLY, 0);`:创建文件节点。
7. `inode = file->f_path.dentry->d_inode;`:获取文件节点。
8. `err = kernel_write(file, data, size, &file->f_pos);`:将文件数据写入文件。
9. `inode->i_mode = S_IRUSR | S_IWUSR | S_IRGRP | S_IROTH;`:设置文件权限。
10. `fput(file);`:关闭文件。
11. `deactivate_super(sb);`:卸载根文件系统。
12. `free_cpio(&cpio);`:释放 `cpio_data` 结构体。
13. `return err;`:返回错误码。
Linux的文件系统中iterate_shared函数如何实现
`iterate_shared()` 函数是 Linux 文件系统中的一个遍历目录项的函数,它的作用是遍历一个目录下所有的目录项。具体实现如下:
```c
int iterate_shared(struct file *file, struct dir_context *ctx)
{
struct inode *inode = file_inode(file);
struct super_block *sb = inode->i_sb;
struct dentry *parent = file->f_path.dentry;
struct dentry *dentry, *prev;
int err = 0;
spin_lock(&parent->d_lock);
dentry = parent->d_subdirs;
if (dentry)
dget(dentry);
spin_unlock(&parent->d_lock);
while (dentry) {
if (ctx->pos >= ctx->count)
break;
if (!dentry->d_inode)
continue;
if (dentry->d_name.len == 1 && dentry->d_name.name[0] == '.')
goto next;
if (dentry->d_name.len == 2 && dentry->d_name.name[0] == '.' &&
dentry->d_name.name[1] == '.')
goto next;
prev = ctx->pos;
err = dir_emit(ctx, dentry->d_name.name, dentry->d_name.len,
dentry->d_inode->i_ino, dentry->d_inode->i_mode);
if (err)
break;
if (ctx->pos != prev) {
spin_lock(&dentry->d_lock);
if (d_unhashed(dentry)) {
spin_unlock(&dentry->d_lock);
goto next;
}
if (dentry->d_inode)
inotify_inode_queue_event(dentry, IN_ACCESS, 0);
spin_unlock(&dentry->d_lock);
}
next:
spin_lock(&parent->d_lock);
dentry = dentry->d_u.d_child.d_next;
if (dentry)
dget(dentry);
spin_unlock(&prev->d_lock);
dput(prev);
}
if (dentry) {
spin_lock(&parent->d_lock);
dput(dentry);
spin_unlock(&parent->d_lock);
}
return err;
}
```
该函数有两个参数,第一个参数是一个 `file` 结构体,代表要遍历的目录文件。第二个参数是一个 `dir_context` 结构体,包含了遍历过程中需要的一些信息,如遍历到的目录项数量、目录项的位置等。
函数的主要实现是通过遍历目录项链表,获取每个目录项的名称和 inode 号,并将这些信息传递给回调函数 `dir_emit()`,由回调函数进行处理。在遍历过程中,会跳过当前目录和上级目录,同时会对每个目录项进行一些额外的处理,如判断目录项是否已经被卸载、是否需要发送事件通知等。
最后,该函数会返回一个错误码,表示遍历过程中是否出现了错误,如内存分配失败、回调函数返回错误等。
阅读全文
相关推荐
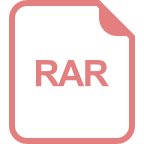
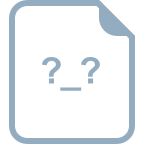
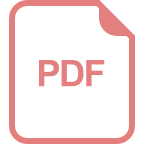
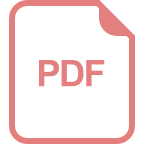
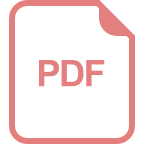
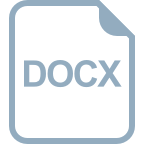
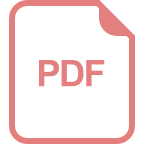
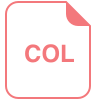
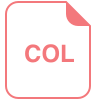
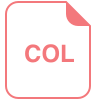
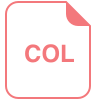



