如何有效地将从输入源读取的字符串转换并存储到字符数组中?
时间: 2024-11-10 22:14:03 浏览: 5
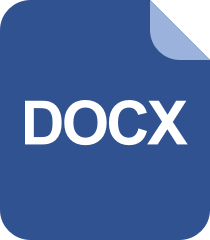
ABB机器人在RobotStudio中进行数字与字符串相互转换的具体方法.docx
在C语言中,避免`scanf()`和`gets()`函数的安全隐患,可以使用`fgets()`来安全地读取字符串。以下是一个示例:
```c
#include <stdio.h>
#include <string.h> // 引入fgets() 和 strlen()
#define MAX_LEN 100 // 定义字符串的最大长度
int main() {
char str[MAX_LEN]; // 定义字符数组来存储字符串
FILE *file = stdin; // 使用标准输入作为文件指针
if (fgets(str, sizeof(str), file) != NULL) { // 使用fgets读取字符串,直到换行符
str[strlen(str) - 1] = '\0'; // 去除可能存在的换行符
printf("Read string: %s\n", str);
} else {
printf("Error reading input.\n");
}
return 0;
}
```
这段代码首先定义了一个字符数组`str`,然后通过`fgets()`函数从标准输入流(`stdin`)读取字符串,直到遇到换行符或达到数组长度。最后,它会移除字符串结束时可能存在的换行符。
要统计字符串中字符出现的次数,你可以参照第2个引用的内容[^1],使用一个计数数组和遍历字符串的方法:
```c
#include <stdio.h>
int main() {
const int CHAR_COUNT = 26;
int count[CHAR_COUNT] = {0}; // 初始化字符计数数组
char str[11];
printf("Enter a string: ");
fgets(str, sizeof(str), stdin);
// 去除换行符
str[strlen(str) - 1] = '\0';
for (size_t i = 0; str[i] != '\0'; i++) {
int index = str[i] - 'a'; // 获取ASCII字符对应的数组下标
count[index]++;
}
for (int i = 0; i < CHAR_COUNT; i++) {
if (count[i] > 0) {
printf("Character '%c' appears %d times.\n", i + 'a', count[i]);
}
}
return 0;
}
```
这个程序会统计用户输入字符串中每个小写字母出现的次数。
阅读全文
相关推荐
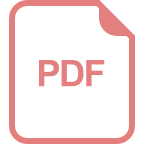
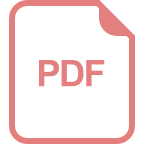
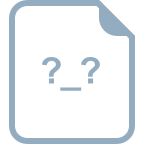
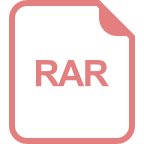
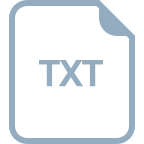
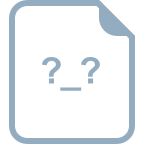
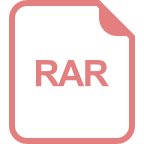
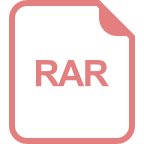
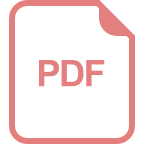
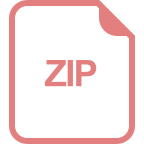
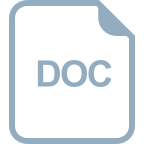






