如何遍历unordered map
时间: 2023-11-07 15:05:53 浏览: 82
要遍历一个unordered map,你可以使用迭代器来访问每个键值对。
以下是一个示例代码,演示如何遍历unordered map并打印其键和值:
```cpp
#include <iostream>
#include <unordered_map>
int main() {
std::unordered_map<std::string, int> myMap = {{"A", 1}, {"B", 2}, {"C", 3}};
// 使用auto来声明迭代器类型
for (auto it = myMap.begin(); it != myMap.end(); ++it) {
std::cout << "Key: " << it->first << ", Value: " << it->second << std::endl;
}
return 0;
}
```
该代码将输出:
```
Key: A, Value: 1
Key: B, Value: 2
Key: C, Value: 3
```
相关问题
怎么使用std::foreach遍历unorderedmap
可以使用C++11的范围for循环或者std::for_each来遍历unordered_map。下面是两种方法的示例:
1. C++11的范围for循环
```cpp
#include <iostream>
#include <unordered_map>
int main()
{
std::unordered_map<std::string, int> mymap = {{"apple", 1}, {"banana", 2}, {"cherry", 3}};
for (auto& pair : mymap) {
std::cout << pair.first << ": " << pair.second << '\n';
}
return 0;
}
```
输出:
```cpp
apple: 1
banana: 2
cherry: 3
```
2. std::for_each
```cpp
#include <iostream>
#include <unordered_map>
#include <algorithm>
int main()
{
std::unordered_map<std::string, int> mymap = {{"apple", 1}, {"banana", 2}, {"cherry", 3}};
std::for_each(mymap.begin(), mymap.end(), [](const auto& pair) {
std::cout << pair.first << ": " << pair.second << '\n';
});
return 0;
}
```
输出:
```cpp
apple: 1
banana: 2
cherry: 3
```
遍历unordered_map
遍历unordered_map可以通过以下几种方式实现:
1. 使用迭代器进行遍历:可以通过遍历unordered_map的迭代器来访问其中的键和值。示例代码如下:
```cpp
unordered_map<int, string> myMap;
// 添加键值对到unordered_map中
for (auto it = myMap.begin(); it != myMap.end(); ++it) {
// 访问键和值
cout << it->first << " " << it->second << endl;
}
```
2. 使用范围-based for循环进行遍历:可以使用范围-based for循环来遍历unordered_map中的键值对。示例代码如下:
```cpp
unordered_map<int, string> myMap;
// 添加键值对到unordered_map中
for (const auto &kv : myMap) {
// 访问键和值
cout << kv.first << " " << kv.second << endl;
}
```
3. 使用find函数进行遍历:可以使用find函数来查找unordered_map中的键,并通过键来访问对应的值。示例代码如下:
```cpp
unordered_map<int, string> myMap;
// 添加键值对到unordered_map中
for (int key = 0; key < 10; ++key) {
// 使用find函数查找键
auto it = myMap.find(key);
if (it != myMap.end()) {
// 访问值
cout << it->second << endl;
}
}
```
以上是遍历unordered_map的几种常用方式。根据不同的需求,选择适合的遍历方式即可。
阅读全文
相关推荐
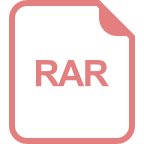
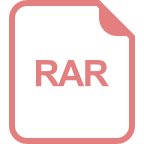
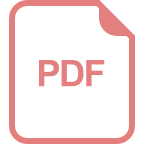













