vue 跑马灯 横向滚动 无缝连接
时间: 2023-07-11 16:20:06 浏览: 146
要实现 Vue 跑马灯横向滚动并且无缝连接,可以采用以下步骤:
1. 在 Vue 组件中编写 HTML 和 CSS,用于显示滚动区域和滚动内容。
2. 在 Vue 组件的 data 中定义一个数组,用于存储滚动内容的数据。
3. 在 Vue 组件的 mounted 钩子中,使用定时器和 CSS3 动画实现滚动效果。
4. 在滚动到最后一个元素时,将第一个元素移动到最后一个元素的后面,从而实现无缝连接的效果。
下面是一个简单的示例代码,供你参考:
```html
<template>
<div class="scroll-container">
<div class="scroll-content">
<div v-for="(item, index) in items" :key="index" class="scroll-item">
{{ item }}
</div>
</div>
</div>
</template>
<script>
export default {
data() {
return {
items: ['item1', 'item2', 'item3', 'item4', 'item5']
};
},
mounted() {
const container = this.$el.querySelector('.scroll-container');
const content = this.$el.querySelector('.scroll-content');
const items = this.$el.querySelectorAll('.scroll-item');
let index = 0;
setInterval(() => {
const currentItem = items[index];
const nextItem = items[(index + 1) % items.length];
content.style.transform = `translateX(-${currentItem.offsetLeft}px)`;
container.style.width = `${currentItem.offsetWidth}px`;
setTimeout(() => {
currentItem.classList.add('scroll-item-hidden');
nextItem.classList.remove('scroll-item-hidden');
if (index === items.length - 1) {
items[0].classList.remove('scroll-item-hidden');
}
index = (index + 1) % items.length;
}, 500);
}, 3000);
}
};
</script>
<style>
.scroll-container {
overflow: hidden;
}
.scroll-content {
display: flex;
transition: transform 0.5s ease;
}
.scroll-item {
flex: none;
margin-right: 20px;
padding: 10px;
background-color: #eee;
}
.scroll-item-hidden {
display: none;
}
</style>
```
在这个示例中,我们定义了一个包含 5 个元素的数组 items,用于存储滚动内容的数据。在组件的 mounted 钩子中,我们使用了 setInterval 和 setTimeout 方法实现了滚动和无缝连接的效果。CSS 方面,我们使用了 flex 布局和 transform 属性实现了滚动的效果,使用了 display 属性实现了无缝连接的效果。
阅读全文
相关推荐
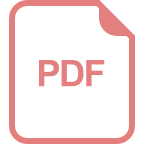


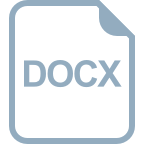
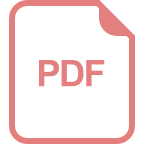
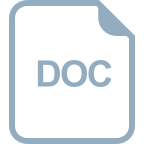
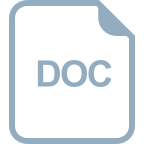

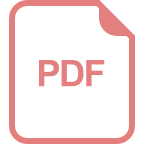
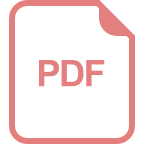
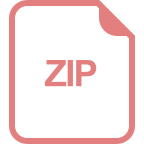