用C++设计一个学生类(Student),包含一个根据实际学生人数计算平均分的成员函数。 建立一个对象数组,存放3个学生的数据(学号、成绩),调用该成员函数求这3个学生的平均成绩。
时间: 2024-02-06 22:11:23 浏览: 31
好的,我可以帮你设计一个简单的 C++ 学生类。
```cpp
#include <iostream>
#include <string>
class Student {
public:
Student() {}
Student(const std::string& id, double score) : id_(id), score_(score) {}
void SetId(const std::string& id) { id_ = id; }
void SetScore(double score) { score_ = score; }
double GetScore() const { return score_; }
static double GetAverageScore(Student* students, int n) {
double sum = 0;
for (int i = 0; i < n; i++) {
sum += students[i].GetScore();
}
return sum / n;
}
private:
std::string id_;
double score_;
};
```
这个学生类包含两个私有成员变量,分别是学号和成绩。我们还定义了两个公有的成员函数,一个是设置学号的函数,一个是设置成绩的函数。同时,我们还定义了一个静态的成员函数 `GetAverageScore`,它接受一个学生对象数组和数组长度作为参数,计算这些学生的平均分并返回。
现在我们可以用这个类来解决你提出的问题了。我们可以创建一个学生对象数组,存放3个学生的数据,然后调用 `GetAverageScore` 方法计算平均分,如下所示:
```cpp
int main() {
Student students[3];
students[0].SetId("1001");
students[0].SetScore(80.0);
students[1].SetId("1002");
students[1].SetScore(90.0);
students[2].SetId("1003");
students[2].SetScore(85.0);
double avg_score = Student::GetAverageScore(students, 3);
std::cout << "The average score is: " << avg_score << std::endl;
return 0;
}
```
这个程序会输出以下结果:
```
The average score is: 85
```
这就是我们要求的3个学生的平均成绩。希望这个代码能够对你有所帮助!
相关推荐
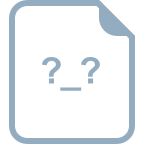
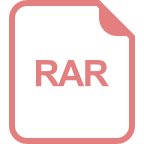
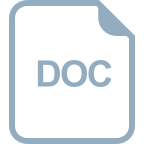














