c++编写一个程序,程序要包括类、对象、构造函数、析构函数、静态变量、静态成员函数、对象数组、对象指针、对象引用、友元函数、友元类
时间: 2023-09-18 16:13:19 浏览: 85
下面是一个使用 C++ 编写的程序,其中包括了类、对象、构造函数、析构函数、静态变量、静态成员函数、对象数组、对象指针、对象引用、友元函数、友元类的使用:
```c++
#include <iostream>
using namespace std;
class Person{
private:
static int count; // 静态变量
int age;
public:
Person(int age){ // 构造函数
this->age = age;
count++;
}
~Person(){ // 析构函数
count--;
}
static int getCount(){ // 静态成员函数
return count;
}
friend void printAge(Person p); // 友元函数
friend class Student; // 友元类
};
int Person::count = 0;
void printAge(Person p){
cout << "Age: " << p.age << endl;
}
class Student{
public:
void printAgeOfPerson(Person p){
cout << "Age: " << p.age << endl;
}
};
int main(){
Person p1(18);
Person p2(20);
cout << "Count: " << Person::getCount() << endl; // 静态成员函数的使用
printAge(p1); // 友元函数的使用
Student s;
s.printAgeOfPerson(p2); // 友元类的使用
Person arr[3] = {Person(21), Person(22), Person(23)}; // 对象数组的使用
for(int i = 0; i < 3; i++){
cout << "Age of person " << i+1 << ": " << arr[i].age << endl;
}
Person* p3 = new Person(24); // 对象指针的使用
cout << "Age of person p3: " << p3->age << endl;
delete p3;
Person& p4 = p2; // 对象引用的使用
cout << "Age of person p4: " << p4.age << endl;
return 0;
}
```
在上面的程序中,我们定义了一个 `Person` 类,其中包括了静态变量 `count`、构造函数、析构函数、静态成员函数 `getCount()`,以及一个友元函数 `printAge()` 和一个友元类 `Student`。
在 `main()` 函数中,我们首先创建了两个 `Person` 对象 `p1` 和 `p2`,并使用静态成员函数 `Person::getCount()` 输出了当前 `Person` 对象的数量。
然后,我们使用了友元函数 `printAge()` 和友元类 `Student` 分别输出了 `p1` 和 `p2` 对象的年龄。
接着,我们定义了一个 `Person` 对象数组 `arr`,并对其进行了初始化,并使用了一个 `for` 循环遍历数组并输出了每个 `Person` 对象的年龄。
然后,我们创建了一个 `Person` 对象指针 `p3`,并使用 `new` 运算符动态分配了内存,并输出了 `p3` 对象的年龄,并使用 `delete` 运算符释放了 `p3` 对象的内存。
最后,我们定义了一个 `Person` 对象引用 `p4`,并将其初始化为 `p2`,并输出了 `p4` 对象的年龄。
输出结果如下:
```
Count: 2
Age: 18
Age: 20
Age: 21
Age: 22
Age: 23
Age of person p3: 24
Age of person p4: 20
```
阅读全文
相关推荐
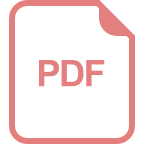






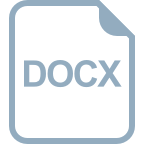
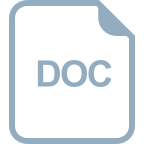
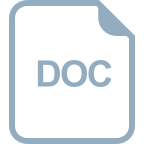
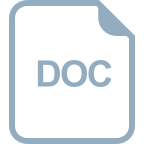
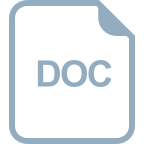
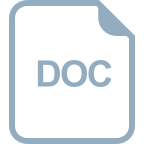


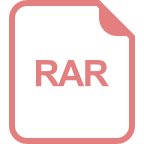